일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
- 프로그래머스
- 자연어처리
- hackerrank
- 맥북
- SW Expert Academy
- ubuntu
- 편스토랑
- 파이썬
- Git
- leetcode
- gs25
- AI 경진대회
- 백준
- 더현대서울 맛집
- ChatGPT
- 우분투
- 편스토랑 우승상품
- github
- 프로그래머스 파이썬
- 데이콘
- Docker
- 코로나19
- Baekjoon
- Real or Not? NLP with Disaster Tweets
- 캐치카페
- 금융문자분석경진대회
- dacon
- PYTHON
- programmers
- Kaggle
- Today
- Total
목록
반응형
PYTHON (460)
솜씨좋은장씨
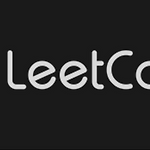
Write a program to check whether a given number is an ugly number. Ugly numbers are positive numbers whose prime factors only include 2, 3, 5. Example 1: Input: 6 Output: true Explanation: 6 = 2 × 3 Example 2: Input: 8 Output: true Explanation: 8 = 2 × 2 × 2 Example 3: Input: 14 Output: false Explanation: 14 is not ugly since it includes another prime factor 7. Note: 1 is typically treated as an..
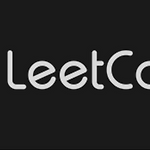
Given an array nums with n integers, your task is to check if it could become non-decreasing by modifying at most 1 element. We define an array is non-decreasing if nums[i]
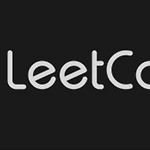
Write a function that reverses a string. The input string is given as an array of characters char[]. Do not allocate extra space for another array, you must do this by modifying the input array in-place with O(1) extra memory. You may assume all the characters consist of printable ascii characters. Example 1: Input: ["h","e","l","l","o"] Output: ["o","l","l","e","h"] Example 2: Input: ["H","a","..
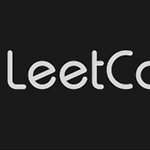
Say you have an array for which the ith element is the price of a given stock on day i. If you were only permitted to complete at most one transaction (i.e., buy one and sell one share of the stock), design an algorithm to find the maximum profit. Note that you cannot sell a stock before you buy one. Example 1: Input: [7,1,5,3,6,4] Output: 5 Explanation: Buy on day 2 (price = 1) and sell on day ..
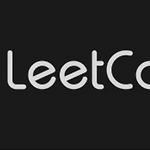
Given a string s and a string t, check if s is subsequence of t. A subsequence of a string is a new string which is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (ie, "ace" is a subsequence of "abcde" while "aec" is not). Follow up: If there are lots of incoming S, say S1, S2, ... , Sk where..
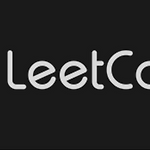
We are given two arrays A and B of words. Each word is a string of lowercase letters. Now, say that word b is a subset of word a if every letter in b occurs in a, including multiplicity. For example, "wrr" is a subset of "warrior", but is not a subset of "world". Now say a word a from A is universal if for every b in B, b is a subset of a. Return a list of all universal words in A. You can retur..
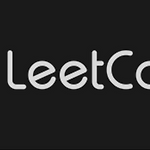
You are given a string representing an attendance record for a student. The record only contains the following three characters: 'A' : Absent. 'L' : Late. 'P' : Present. A student could be rewarded if his attendance record doesn't contain more than one 'A' (absent) or more than two continuous 'L' (late). You need to return whether the student could be rewarded according to his attendance record...
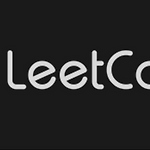
Write a function that takes a string as input and reverse only the vowels of a string. Example 1: Input: "hello" Output: "holle" Example 2: Input: "leetcode" Output: "leotcede" Note: The vowels does not include the letter "y". Solution class Solution(object): def reverseVowels(self, s): s_list = list(s) vowels = [] for i, val in enumerate(s_list): if val in ['a', 'o', 'e', 'i', 'u', 'A', 'O', 'E..
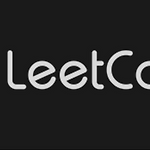
Given an array of integers where 1 ≤ a[i] ≤ n (n = size of array), some elements appear twice and others appear once. Find all the elements of [1, n] inclusive that do not appear in this array. Could you do it without extra space and in O(n) runtime? You may assume the returned list does not count as extra space. Example: Input: [4,3,2,7,8,2,3,1] Output: [5,6] Solution class Solution: def findDi..
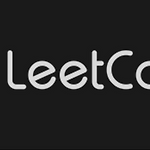
Given a sorted array and a target value, return the index if the target is found. If not, return the index where it would be if it were inserted in order. You may assume no duplicates in the array. Example 1: Input: [1,3,5,6], 5 Output: 2 Example 2: Input: [1,3,5,6], 2 Output: 1 Example 3: Input: [1,3,5,6], 7 Output: 4 Example 4: Input: [1,3,5,6], 0 Output: 0 Solution class Solution: def searchI..
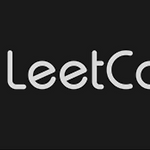
Given a pattern and a string str, find if str follows the same pattern. Here follow means a full match, such that there is a bijection between a letter in pattern and a non-empty word in str. Example 1: Input: pattern = "abba", str = "dog cat cat dog" Output: true Example 2: Input:pattern = "abba", str = "dog cat cat fish" Output: false Example 3: Input: pattern = "aaaa", str = "dog cat cat dog"..
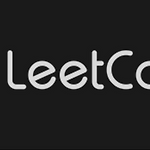
Given two strings s and t, determine if they are isomorphic. Two strings are isomorphic if the characters in s can be replaced to get t. All occurrences of a character must be replaced with another character while preserving the order of characters. No two characters may map to the same character but a character may map to itself. Example 1: Input: s = "egg", t = "add" Output: true Example 2: In..
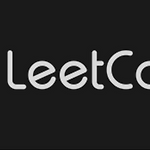
Count the number of segments in a string, where a segment is defined to be a contiguous sequence of non-space characters. Please note that the string does not contain any non-printable characters. Example: Input: "Hello, my name is John" Output: 5 Solution class Solution: def countSegments(self, s: str) -> int: answer = len(s.split()) return answer SOMJANG/CODINGTEST_PRACTICE 1일 1문제 since 2020.0..
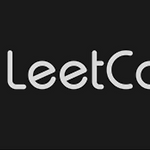
Given a string s and an integer array indices of the same length. The string s will be shuffled such that the character at the ith position moves to indices[i] in the shuffled string. Return the shuffled string. Example 1: Input: s = "codeleet", indices = [4,5,6,7,0,2,1,3] Output: "leetcode" Explanation: As shown, "codeleet" becomes "leetcode" after shuffling. Example 2: Input: s = "abc", indice..
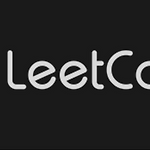
We are given two sentences A and B. (A sentence is a string of space separated words. Each word consists only of lowercase letters.) A word is uncommon if it appears exactly once in one of the sentences, and does not appear in the other sentence. Return a list of all uncommon words. You may return the list in any order. Example 1: Input: A = "this apple is sweet", B = "this apple is sour" Output..
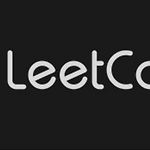
Given two arrays, write a function to compute their intersection. Example 1: Input: nums1 = [1,2,2,1], nums2 = [2,2] Output: [2] Example 2: Input: nums1 = [4,9,5], nums2 = [9,4,9,8,4] Output: [9,4] Note: Each element in the result must be unique. The result can be in any order. Solution class Solution: def intersection(self, nums1: List[int], nums2: List[int]) -> List[int]: return list(set(nums1..
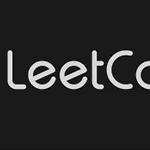
Example 3: Given a date, return the corresponding day of the week for that date. The input is given as three integers representing the day, month and year respectively. Return the answer as one of the following values {"Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday"}. Example 1: Input: day = 31, month = 8, year = 2019 Output: "Saturday" Example 2: Input: day = 18, mo..
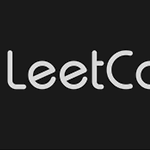
Given an array of numbers nums, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. Example: Input: [1,2,1,3,2,5] Output: [3,5] Note: The order of the result is not important. So in the above example, [5, 3] is also correct. Your algorithm should run in linear runtime complexity. Could you implement it using..
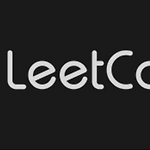
You are given two non-empty linked lists representing two non-negative integers. The most significant digit comes first and each of their nodes contain a single digit. Add the two numbers and return it as a linked list. You may assume the two numbers do not contain any leading zero, except the number 0 itself. Follow up: What if you cannot modify the input lists? In other words, reversing the li..
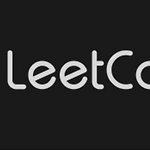
Given an array nums containing n + 1 integers where each integer is between 1 and n (inclusive), prove that at least one duplicate number must exist. Assume that there is only one duplicate number, find the duplicate one. Example 1: Input: [1,3,4,2,2] Output: 2 Example 2: Input: [3,1,3,4,2] Output: 3 Note: You must not modify the array (assume the array is read only). You must use only constant,..
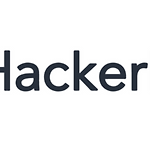
Comparators are used to compare two objects. In this challenge, you'll create a comparator and use it to sort an array. The Player class is provided in the editor below. It has two fields: name : a string. score : an integer. Given an array of n Player objects, write a comparator that sorts them in order of decreasing score. If 2 or more players have the same score, sort those players alphabetic..
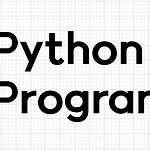
Python으로 프로그래밍을 하다보면 가끔 아래와 같은 오류를 만나는 경우가 있습니다. SyntaxError: Non-ASCII character '\xeb' in file 위의 오류는 코드 내부에 한글 데이터가 포함 되어있을 경우 발생하는 문제입니다. 이걸 해결하기 위해서 코드에 있는 한글을 다 삭제하거나 영어로 번역하지 않아도 됩니다. 해결방법 #-*- coding:utf-8 -*- 해당 코드파일의 코드 맨 윗줄에 위의 코드를 추가해주고 다시 실행해보면 정상적으로 작동하는 것을 볼 수 있습니다! 읽어주셔서 감사합니다!
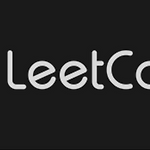
Given an array containing n distinct numbers taken from 0, 1, 2, ..., n, find the one that is missing from the array. Example 1: Input: [3,0,1] Output: 2 Example 2: Input: [9,6,4,2,3,5,7,0,1] Output: 8 Note: Your algorithm should run in linear runtime complexity. Could you implement it using only constant extra space complexity? Solution class Solution: def missingNumber(self, nums: List[int]) -..
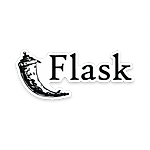
Flask로 웹페이지를 만들면서 npm 라이브러리를 사용해야하는 경우 다음과 같은 방법으로 사용하면 됩니다. 다음의 방법은 node.js가 미리 설치되어있다는 가정 하에 가능합니다. Mac을 사용하고 brew를 설치하셨다면 $ brew install node 위의 명령어를 활용하여 설치하여 줍니다. 먼저 Flask 프로젝트의 static 디렉토리로 이동합니다. $ cd static 그 다음 다음의 명령어를 활용하여 npm 프로젝트로 initialize 시켜줍니다. $ npm init 그 다음 npm라이브러리 중 사용을 희망하는 라이브러리를 설치하고 저장합니다. 예시로는 dom-inspector라는 오픈소스 라이브러리를 예시로 들겠습니다. $ npm install dom-inspector --save 완료..
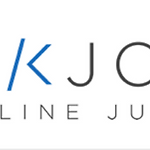
1일 1문제 107일차! 오늘의 문제는 백준의 오르막 수 입니다. 11057번: 오르막 수 오르막 수는 수의 자리가 오름차순을 이루는 수를 말한다. 이때, 인접한 수가 같아도 오름차순으로 친다. 예를 들어, 2234와 3678, 11119는 오르막 수이지만, 2232, 3676, 91111은 오르막 수가 아니다. 수� www.acmicpc.net Solution inputNum = int(input()) nc = [[0]*10 for _ in range(inputNum+1)] ans, mod = 0, 10007 for i in range(0, 10): nc[1][i] = 1 for i in range(2, inputNum+1): nc[i][0] = nc[i-1][0] for j in range(1, 10..
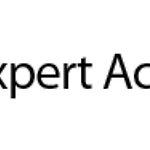
1일 1문제 106일차! 오늘의 문제는 SW Expert Academy 새샘이의 7-3-5 게임입니다. SW Expert Academy SW 프로그래밍 역량 강화에 도움이 되는 다양한 학습 컨텐츠를 확인하세요! swexpertacademy.com Solution from itertools import combinations T = int(input()) for i in range(T): numbers = list(set(map(int, input().split(' ')))) comb = combinations(numbers, 3) comb_sum = [sum(cmb) for cmb in comb] comb_sum = list(set(comb_sum)) comb_sort = sorted(comb_sum, ..
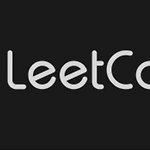
Given a non-empty array of digits representing a non-negative integer, plus one to the integer. The digits are stored such that the most significant digit is at the head of the list, and each element in the array contain a single digit. You may assume the integer does not contain any leading zero, except the number 0 itself. Example 1: Input: [1,2,3] Output: [1,2,4] Explanation: The array repres..
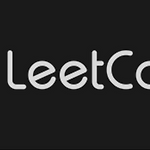
Implement int sqrt(int x). Compute and return the square root of x, where x is guaranteed to be a non-negative integer. Since the return type is an integer, the decimal digits are truncated and only the integer part of the result is returned. Example 1: Input: 4 Output: 2 Example 2: Input: 8 Output: 2 Explanation: The square root of 8 is 2.82842..., and since the decimal part is truncated, 2 is ..
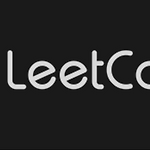
Implementatoiwhich converts a string to an integer. The function first discards as many whitespace characters as necessary until the first non-whitespace character is found. Then, starting from this character, takes an optional initial plus or minus sign followed by as many numerical digits as possible, and interprets them as a numerical value. The string can contain additional characters after ..
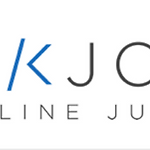
1일 1문제! 오늘은 1일 1문제의 100일차!!!!!!! 백준의 계단 오르기입니다. 2579번: 계단 오르기 계단 오르기 게임은 계단 아래 시작점부터 계단 꼭대기에 위치한 도착점까지 가는 게임이다. 과 같이 각각의 계단에는 일정한 점수가 쓰여 있는데 계단을 밟으면 그 계단에 쓰여 있는 점 www.acmicpc.net Solution inputNum = int(input()) stairScores = [] for i in range(inputNum): inputScore = int(input()) stairScores.append(inputScore) maxScore = [0] * inputNum for i in range(inputNum): if i == 0: maxScore[0] = stairScor..