일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- PYTHON
- ChatGPT
- AI 경진대회
- gs25
- 자연어처리
- 편스토랑 우승상품
- Kaggle
- 맥북
- ubuntu
- 더현대서울 맛집
- Baekjoon
- Docker
- 데이콘
- Git
- 우분투
- leetcode
- 파이썬
- Real or Not? NLP with Disaster Tweets
- 프로그래머스
- 코로나19
- hackerrank
- programmers
- 백준
- dacon
- 금융문자분석경진대회
- 캐치카페
- 편스토랑
- 프로그래머스 파이썬
- SW Expert Academy
- github
- Today
- Total
솜씨좋은장씨
[Programmers] 2023 KAKAO BLIND RECRUITMENT - 개인정보 수집 유효기간 (Python) 본문
[Programmers] 2023 KAKAO BLIND RECRUITMENT - 개인정보 수집 유효기간 (Python)
솜씨좋은장씨 2023. 1. 26. 13:26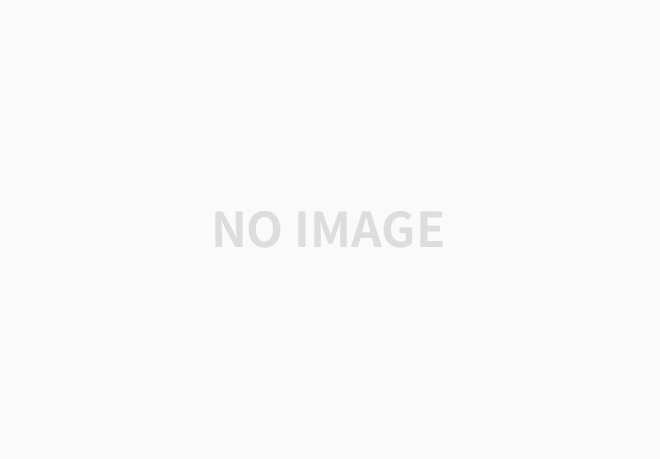
코딩 1일 1문제! 오늘의 문제는 Programmers 의 2023 KAKAO BLIND RECRUITMENT 개인정보 수집 유효기간 입니다.
프로그래머스
코드 중심의 개발자 채용. 스택 기반의 포지션 매칭. 프로그래머스의 개발자 맞춤형 프로필을 등록하고, 나와 기술 궁합이 잘 맞는 기업들을 매칭 받으세요.
programmers.co.kr
👨🏻💻 문제 풀이
1. 입력 받은 약관 리스트를 dictionary 형태로 변환하는 함수 작성
- 각 term 은 "약관명 기간" 형식으로 작성되어있으므로 공백 기준으로 split 하고
- split 한 값의 앞의 값을 dictionary 의 key 로 뒤의 값을 int 형식으로 변환하여 value 값으로 넣어주었습니다.
def convert_terms_to_dict(terms):
terms_dict = {}
for term in terms:
term_name, term_time = term.split()
terms_dict[term_name] = int(term_time)
return terms_dict
2. 문자열로 된 날짜 데이터를 datetime 객체로 변환하는 함수 작성
- datetime 의 strptime 활용
- 날짜 문자열 형식이 yyyy.mm.dd 형식이므로 strptime 의 인자로 %Y.%m.%d 작성
from datetime import datetime
def convert_str_to_datetime(date_string):
return datetime.strptime(date_string, "%Y.%m.%d")
2022.01.19 - [Programming/Python] - [Python] datetime의 strptime을 활용하여 시간과 시간 사이의 차이 구하는 방법
[Python] datetime의 strptime을 활용하여 시간과 시간 사이의 차이 구하는 방법
개발을 하다 보면 종종 특정 시간과 특정 시간 사이에 얼마나 많은 시간이 흘렀는지 구해야 할 경우가 있습니다. 직접 시간을 계산하는 코드를 작성해도 되지만 2시 39분 -> 5시 25분 까지 얼마나
somjang.tistory.com
3. 만료일을 구하는 함수 작성
- dateutil 의 relativedelta 활용
from dateutil.relativedelta import relativedelta
def get_expired_date(collection_date, term_dict, term_type):
return collection_date + relativedelta(months=term_dict[term_type])
4. 위에서 작성한 함수를 활용하여 개인정보 만료 여부를 확인하는 함수 작성
- 인자로 받은 privacy 는 "수집일자 약관명" 형식으로 되어있으므로 공백 기준으로 split 하여 사용
- 수집일자 문자열을 2번에서 만든 함수를 활용하여 datetime 객체로 변환
- datetime 객체로 변환한 값을 3번에서 만든 함수를 활용하여 개인정보 만료일을 계산
- 마지막으로 오늘 날짜와 만료일을 비교하여 오늘 날짜가 만료일 보다 같거나 크다면 True 를 return 하도록 함
- 그렇지 않을 경우에는 False return
def check_privacy_term_expired(today, privacy, term_dict):
is_expired = False
privacy_collection_date, term_type = privacy.split()
privacy_collection_date = convert_str_to_datetime(
date_string=privacy_collection_date
)
expired_date = get_expired_date(
collection_date=privacy_collection_date,
term_dict=term_dict, term_type=term_type
)
if today >= expired_date:
is_expired = True
return is_expired
5. 위 함수들을 활용하여 최종 정답 solution 함수 작성
- 오늘 날짜를 2번에서 만든 함수를 활용하여 datetime 객체로 변환
- 약관 리스트를 1번에서 만든 함수를 활용하여 dictionary 형태로 변환
- 개인정보 리스트에서 enumerate 를 활용하여 하나씩 꺼내옴 ( 이때 시작 idx를 1로 설정 )
- 꺼내온 개인정보를 4번에서 만든 함수를 활용하여 개인정보 만료 여부 체크
- 만료되었다면 정답 리스트에 idx 를 넣어줌
- 마지막으로 정답 리스트를 return 하면 끝
def solution(today, terms, privacies):
answer = []
today = convert_str_to_datetime(date_string=today)
term_dict = convert_terms_to_dict(terms=terms)
for privacy_idx, privacy in enumerate(privacies, start=1):
is_expired = check_privacy_term_expired(
today=today, privacy=privacy, term_dict=term_dict
)
if is_expired:
answer.append(privacy_idx)
return answer
전체 코드는 아래를 참고해주세요.
👨🏻💻 코드 ( Solution )
from datetime import datetime
from dateutil.relativedelta import relativedelta
def convert_terms_to_dict(terms):
terms_dict = {}
for term in terms:
term_name, term_time = term.split()
terms_dict[term_name] = int(term_time)
return terms_dict
def convert_str_to_datetime(date_string):
return datetime.strptime(date_string, "%Y.%m.%d")
def get_expired_date(collection_date, term_dict, term_type):
return collection_date + relativedelta(months=term_dict[term_type])
def check_privacy_term_expired(today, privacy, term_dict):
is_expired = False
privacy_collection_date, term_type = privacy.split()
privacy_collection_date = convert_str_to_datetime(
date_string=privacy_collection_date
)
expired_date = get_expired_date(
collection_date=privacy_collection_date,
term_dict=term_dict, term_type=term_type
)
if today >= expired_date:
is_expired = True
return is_expired
def solution(today, terms, privacies):
answer = []
today = convert_str_to_datetime(date_string=today)
term_dict = convert_terms_to_dict(terms=terms)
for privacy_idx, privacy in enumerate(privacies, start=1):
is_expired = check_privacy_term_expired(
today=today, privacy=privacy, term_dict=term_dict
)
if is_expired:
answer.append(privacy_idx)
return answer
GitHub - SOMJANG/CODINGTEST_PRACTICE: 1일 1문제 since 2020.02.07
1일 1문제 since 2020.02.07. Contribute to SOMJANG/CODINGTEST_PRACTICE development by creating an account on GitHub.
github.com
'Programming > 코딩 1일 1문제' 카테고리의 다른 글
[BaekJoon] 27294번 : 몇개고? (Python) (0) | 2023.01.30 |
---|---|
[Programmers] 최빈값 구하기 (Python) (2) | 2023.01.28 |
[BaekJoon] 23809번 : 골뱅이 찍기 - 돌아간 ㅈ (Python) (1) | 2023.01.25 |
[BaekJoon] 26561번 : Population (Python) (0) | 2023.01.24 |
[BaekJoon] 23810번 : 골뱅이 찍기 - ㅋ (Python) (0) | 2023.01.23 |