일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 파이썬
- Real or Not? NLP with Disaster Tweets
- dacon
- 데이콘
- 자연어처리
- Git
- leetcode
- 금융문자분석경진대회
- Docker
- SW Expert Academy
- ubuntu
- hackerrank
- 프로그래머스
- PYTHON
- 우분투
- 캐치카페
- Kaggle
- 백준
- github
- programmers
- AI 경진대회
- Baekjoon
- 프로그래머스 파이썬
- 편스토랑
- 편스토랑 우승상품
- gs25
- 더현대서울 맛집
- 맥북
- 코로나19
- ChatGPT
- Today
- Total
솜씨좋은장씨
[HackerRank] Jumping on the Clouds (Python) 본문
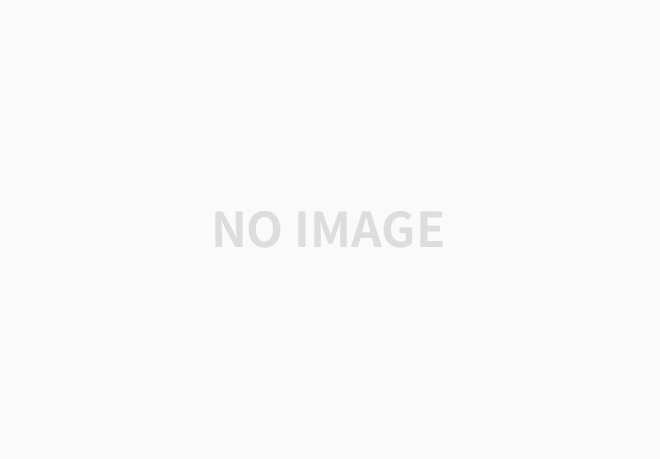
Emma is playing a new mobile game that starts with consecutively numbered clouds. Some of the clouds are thunderheads and others are cumulus. She can jump on any cumulus cloud having a number that is equal to the number of the current cloud plus 1 or 2. She must avoid the thunderheads. Determine the minimum number of jumps it will take Emma to jump from her starting postion to the last cloud. It is always possible to win the game.
For each game, Emma will get an array of clouds numbered 0 if they are safe or 1 if they must be avoided.
For example, c = [ 0, 1, 0, 0, 0, 1, 0 ] indexed from 0. . .6. The number on each cloud is its index in the list so she must avoid the clouds at indexes 1 and 5. She could follow the following two paths: 0 → 2 → 4 → 6 or 0 → 2 → 3 → 4 → 6. The first path takes 3 jumps while the second takes 4.
Function Description
Complete the jumpingOnClouds function in the editor below. It should return the minimum number of jumps required, as an integer.
jumpingOnClouds has the following parameter(s):
- c: an array of binary integers
Input Format
The first line contains an integer n, the total number of clouds. The second line contains n space-separated binary integers describing clouds c [ i ] where 0 <= i < n.
Constraints
- 2 <= n <= 100
- c [ i ] { 0, 1 }
- c [ 0 ] = c [ n - 1 ] = 0
Output Format
Print the minimum number of jumps needed to win the game.
Sample Input 0
7
0 0 1 0 0 1 0
Sample Output 0
4
Explanation 0:
Emma must avoid c [ 2 ] and c [ 5 ]. She can win the game with a minimum of 4 jumps:
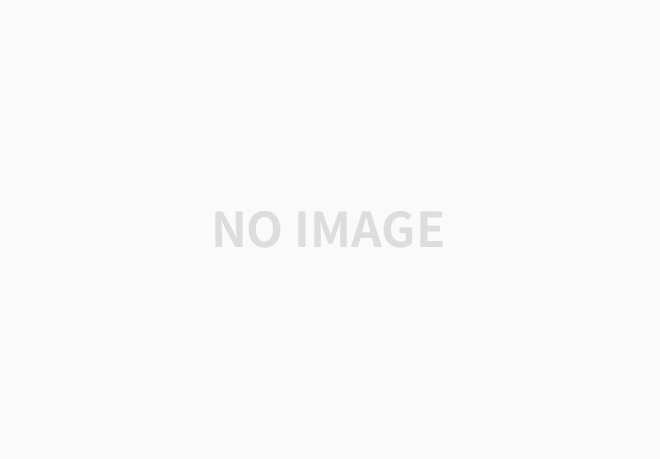
Sample Input 1
6
0 0 0 0 1 0
Sample Output 1
3
Explanation 1:
The only thundercloud to avoid is c [ 4 ]. Emma can win the game in 3 jumps:
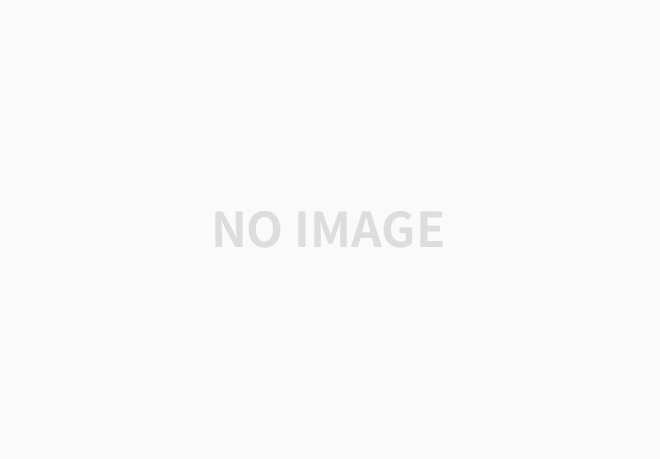
Solution
#!/bin/python3
import math
import os
import random
import re
import sys
# Complete the jumpingOnClouds function below.
def jumpingOnClouds(c):
goal_index = len(c) - 1
check_value = 0
answer = 0
while(check_value < goal_index):
if check_value + 2 <= goal_index and c[check_value + 2] == 0:
check_value = check_value + 2
answer = answer + 1
elif c[check_value + 1] == 0:
check_value = check_value + 1
answer = answer + 1
print(check_value)
return answer
Solution 풀이
먼저 c의 길이를 구하고 1을 빼서 목표 인덱스 값을 구하고
앞에서부터 접근하기 위해 인덱스로 사용할 check_value를 0으로 설정합니다.
한번씩 앞으로 갈때마다 1씩 증가시켜줄 answer도 0으로 설정합니다.
while 반복문을 실행하는데 goal_index 값보다 check_value 값이 크게되면 list의 범위를 초과하므로
check_value < goal_index를 조건으로 넣어주고
먼저 2칸 앞으로 갔을때 그 구름을 밟을 수 있는지 ( 0 ) 없는지 ( 1 ) 를 확인하고
밟을 수 있으면 check_value에 2를 더하고 answer를 하나 증가
밟을 수 없으면 check_value에 1을 더하고 answer를 하나증가 시켜 줍니다.
여기서 근데 2칸 앞으로 가는 경우를 판단할때
check_value의 값이 goal_index - 1일 경우 + 2를 했을때 또 list의 범위를 벗어나므로
먼저 check_value + 2 가 goal_index와 같거나 작은지 먼저 확인해 주어야 합니다.
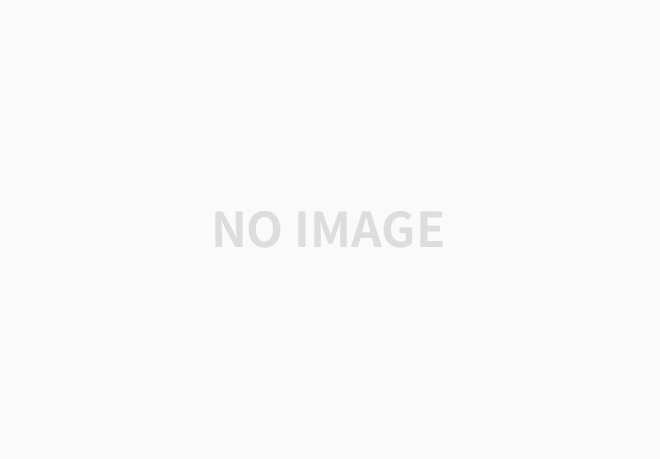
SOMJANG/CODINGTEST_PRACTICE
1일 1문제 since 2020.02.07. Contribute to SOMJANG/CODINGTEST_PRACTICE development by creating an account on GitHub.
github.com
'Programming > 코딩 1일 1문제' 카테고리의 다른 글
[HackerRank] Arrays: Minimum Swaps 2 (Python) (0) | 2020.03.11 |
---|---|
[HackerRank] Sorting: Bubble Sort (Python) (0) | 2020.03.10 |
[HackerRank] Repeated String (Python) (0) | 2020.03.08 |
[HackerRank] Counting Valleys (Python) (0) | 2020.03.07 |
[HackerRank] Sock Merchant (Python) (0) | 2020.03.06 |