일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 우분투
- github
- 백준
- AI 경진대회
- 데이콘
- hackerrank
- 편스토랑 우승상품
- Kaggle
- 맥북
- 자연어처리
- Baekjoon
- 편스토랑
- dacon
- ChatGPT
- programmers
- 파이썬
- Docker
- 프로그래머스
- 캐치카페
- 코로나19
- Git
- 더현대서울 맛집
- PYTHON
- Real or Not? NLP with Disaster Tweets
- gs25
- ubuntu
- 프로그래머스 파이썬
- 금융문자분석경진대회
- SW Expert Academy
- leetcode
- Today
- Total
솜씨좋은장씨
[HackerRank] Sorting: Bubble Sort (Python) 본문
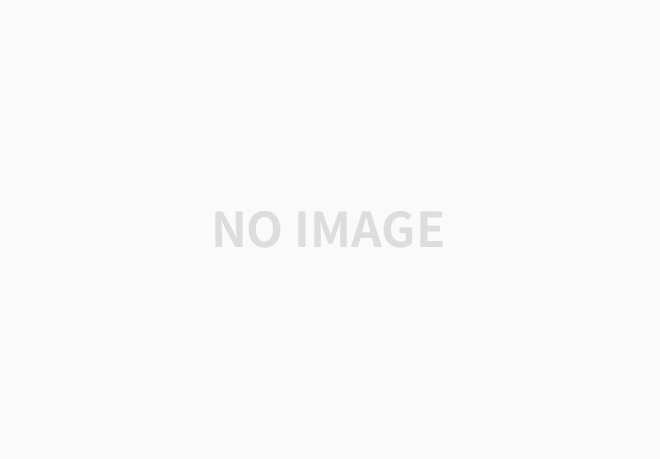
Consider the following version of Bubble Sort:
for (int i = 0; i < n; i++) {
for (int j = 0; j < n - 1; j++) {
// Swap adjacent elements if they are in decreasing order
if (a[j] > a[j + 1]) {
swap(a[j], a[j + 1]);
}
}
}
Given an array of integers, sort the array in ascending order using the Bubble Sort algorithm above. Once sorted, print the following three lines:
- Array is sorted in numSwaps swaps., where numSwaps is the number of swaps that took place.
- First Element: firstElement, where firstElement is the first element in the sorted array.
- Last Element: lastElement, where lastElement is the last element in the sorted array.
Hint: To complete this challenge, you must add a variable that keeps a running tally of all swaps that occur during execution.
For example, given a worst-case but small array to sort: a = [ 6, 4, 1 ] we go through the following steps:
swap a
0 [6,4,1]
1 [4,6,1]
2 [4,1,6]
3 [1,4,6]
It took 3 swaps to sort the array. Output would be
Array is sorted in 3 swaps.
First Element: 1
Last Element: 6
Function Description
Complete the function countSwaps in the editor below. It should print the three lines required, then return.
countSwaps has the following parameter(s):
- a: an array of integers .
Input Format
The first line contains an integer, n, the size of the array a.
The second line contains n space-separated integers a [ i ] .
Constraints
- 2 <= n <= 600
- 1 <= a [ i ] <= 2 x 10^6
Output Format
You must print the following three lines of output:
- Array is sorted in numSwaps swaps., where numSwaps is the number of swaps that took place.
- First Element: firstElement, where firstElement is the first element in the sorted array.
- Last Element: lastElement, where lastElement is the last element in the sorted array.
Sample Input 0
3
1 2 3
Sample Output 0
Array is sorted in 0 swaps.
First Element: 1
Last Element: 3
Explanation 0
The array is already sorted, so 0 swaps take place and we print the necessary three lines of output shown above.
Sample Input 1
3
3 2 1
Sample Output 1
Array is sorted in 3 swaps.
First Element: 1
Last Element: 3
Explanation 1
The array is not sorted, and its initial values are: { 3, 2, 1 }. The following 3 swaps take place:
- { 3, 2, 1 } -> { 2, 3, 1 }
- { 2, 3, 1 } -> { 2, 1, 3 }
- { 2, 1, 3 } -> { 1, 2, 3 }
At this point the array is sorted and we print the necessary three lines of output shown above.
Solution
#!/bin/python3
import math
import os
import random
import re
import sys
# Complete the countSwaps function below.
def countSwaps(a):
count = 0
for i in range(len(a)):
for j in range(len(a)-1):
if a[j] > a[j+1]:
temp = a[j]
a[j] = a[j+1]
a[j+1] = temp
count = count + 1
print("Array is sorted in {} swaps.".format(count))
print("First Element: {}".format(a[0]))
print("Last Element: {}".format(a[-1]))
Solution 풀이
버블 소트를 하면서 카운트하고 나중에 출력시 맨앞 맨뒤의 값 출력을 해주었습니다.
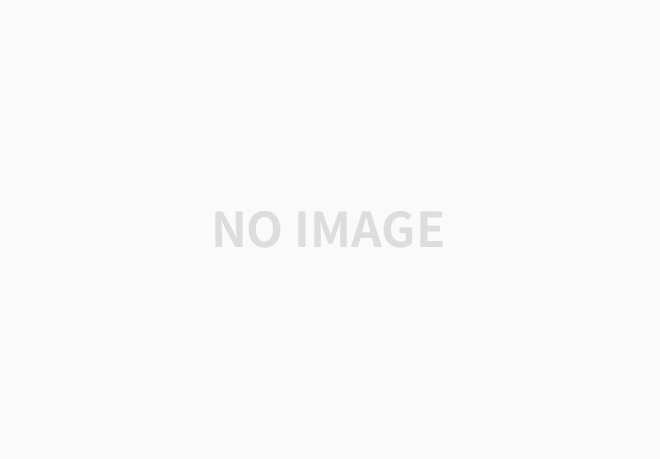
SOMJANG/CODINGTEST_PRACTICE
1일 1문제 since 2020.02.07. Contribute to SOMJANG/CODINGTEST_PRACTICE development by creating an account on GitHub.
github.com
'Programming > 코딩 1일 1문제' 카테고리의 다른 글
[leetCode] 58. Length of Last Word (Python) (2) | 2020.03.12 |
---|---|
[HackerRank] Arrays: Minimum Swaps 2 (Python) (0) | 2020.03.11 |
[HackerRank] Jumping on the Clouds (Python) (0) | 2020.03.09 |
[HackerRank] Repeated String (Python) (0) | 2020.03.08 |
[HackerRank] Counting Valleys (Python) (0) | 2020.03.07 |