일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- Baekjoon
- gs25
- 코로나19
- 더현대서울 맛집
- dacon
- Docker
- github
- Real or Not? NLP with Disaster Tweets
- ChatGPT
- 우분투
- 편스토랑
- programmers
- 맥북
- 프로그래머스 파이썬
- AI 경진대회
- 캐치카페
- 금융문자분석경진대회
- 편스토랑 우승상품
- leetcode
- 데이콘
- ubuntu
- 자연어처리
- hackerrank
- Kaggle
- 프로그래머스
- PYTHON
- 파이썬
- 백준
- Git
- SW Expert Academy
- Today
- Total
솜씨좋은장씨
[leetCode] 929. Unique Email Addresses (Python) 본문
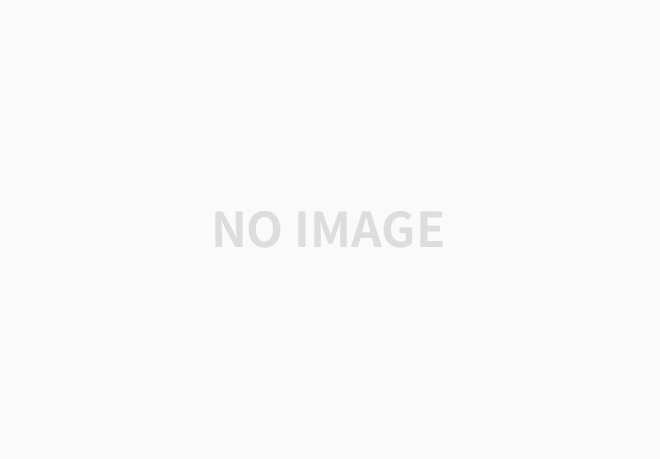
Every email consists of a local name and a domain name, separated by the @ sign.
For example, in alice@leetcode.com, alice is the local name, and leetcode.com is the domain name.
Besides lowercase letters, these emails may contain '.'s or '+'s.
If you add periods ('.') between some characters in the local name part of an email address, mail sent there will be forwarded to the same address without dots in the local name. For example, "alice.z@leetcode.com" and "alicez@leetcode.com" forward to the same email address. (Note that this rule does not apply for domain names.)
If you add a plus ('+') in the local name, everything after the first plus sign will be ignored. This allows certain emails to be filtered, for example m.y+name@email.com will be forwarded to my@email.com. (Again, this rule does not apply for domain names.)
It is possible to use both of these rules at the same time.
Given a list of emails, we send one email to each address in the list. How many different addresses actually receive mails?
Example 1:
Input: ["test.email+alex@leetcode.com","test.e.mail+bob.cathy@leetcode.com","testemail+david@lee.tcode.com"]
Output: 2
Explanation: "testemail@leetcode.com" and "testemail@lee.tcode.com" actually receive mails
Note:
- 1 <= emails[i].length <= 100
- 1 <= emails.length <= 100
- Each emails[i] contains exactly one '@' character.
- All local and domain names are non-empty.
- Local names do not start with a '+' character.
Solution 1
import re
class Solution:
def numUniqueEmails(self, emails: List[str]) -> int:
unique_emails= []
for i in range(len(emails)):
email_front, email_end = emails[i].split('@')
print(email_front, email_end)
email_front = email_front.replace(".", "")
print(email_front, email_end)
email_front_without_localname = email_front.split('+')[0]
email_add = email_front_without_localname + '@' + email_end
if email_add not in unique_emails:
unique_emails.append(email_add)
print(unique_emails)
return len(unique_emails)
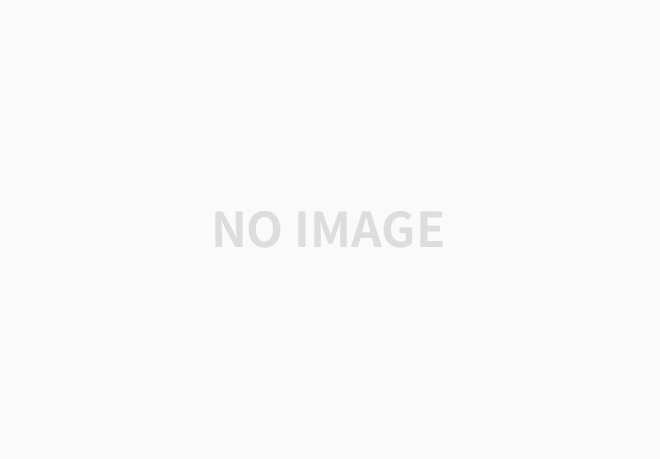
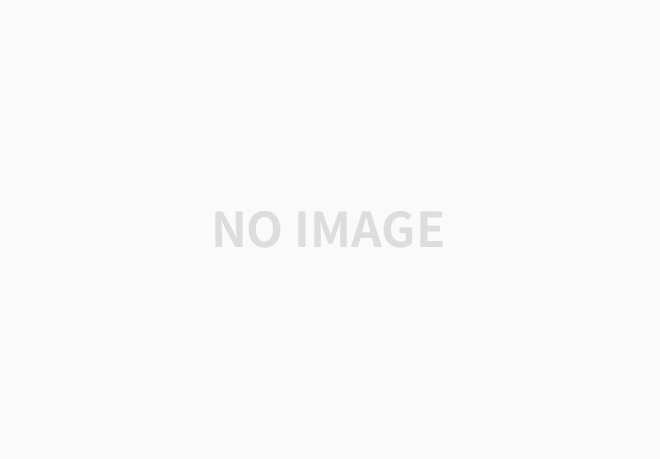
Solution 2
import re
class Solution:
def numUniqueEmails(self, emails: List[str]) -> int:
unique_emails= []
for i in range(len(emails)):
email_regex = re.sub(r'[\+].*[@]', '@', emails[i])
email_front, email_end = email_regex.split('@')
email_front = email_front.replace(".", "")
email_add = email_front + '@' + email_end
if email_add not in unique_emails:
unique_emails.append(email_add)
print(unique_emails)
return len(unique_emails)
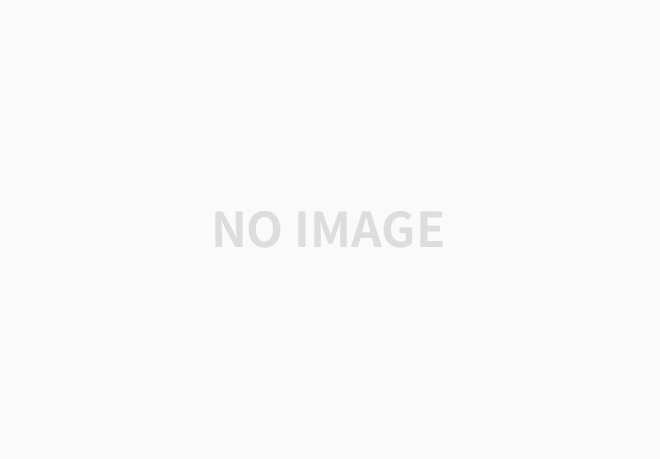
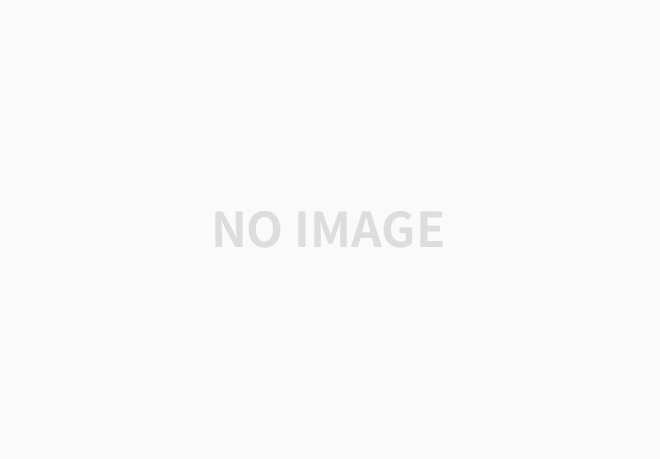
SOMJANG/CODINGTEST_PRACTICE
1일 1문제 since 2020.02.07. Contribute to SOMJANG/CODINGTEST_PRACTICE development by creating an account on GitHub.
github.com
'Programming > 코딩 1일 1문제' 카테고리의 다른 글
[HackerRank] String Manipulation : Alternating Characters (Python) (0) | 2020.03.26 |
---|---|
[HackerRank] Sorting : Mark and Toys (Python) (0) | 2020.03.25 |
[BaeKJoon] 10844번: 쉬운 계단수 (Python) (0) | 2020.03.23 |
[HackerRank] Hash Tables : Ransom Note (Python) (0) | 2020.03.22 |
[SW_Expert_Academy] 4581번 문자열 재구성 프로젝트 (Python) (0) | 2020.03.21 |