Notice
Recent Posts
Recent Comments
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- Docker
- 캐치카페
- github
- programmers
- 자연어처리
- 편스토랑
- 더현대서울 맛집
- leetcode
- Real or Not? NLP with Disaster Tweets
- ubuntu
- 데이콘
- 우분투
- Baekjoon
- 편스토랑 우승상품
- 금융문자분석경진대회
- Git
- 백준
- ChatGPT
- 맥북
- AI 경진대회
- Kaggle
- gs25
- 프로그래머스
- 파이썬
- PYTHON
- 코로나19
- 프로그래머스 파이썬
- SW Expert Academy
- hackerrank
- dacon
Archives
- Today
- Total
솜씨좋은장씨
[leetCode] 859. Buddy Strings (Python) 본문
728x90
반응형
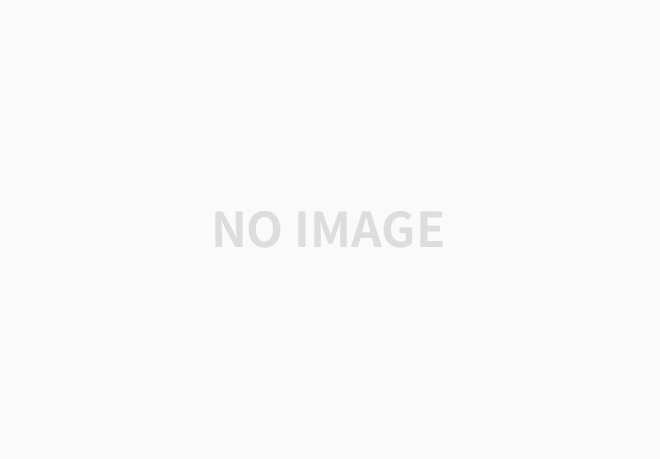
Given two strings A and B of lowercase letters, return true if you can swap two letters in A so the result is equal to B, otherwise, return false.
Swapping letters is defined as taking two indices i and j (0-indexed) such that i != j and swapping the characters at A[i] and A[j]. For example, swapping at indices 0 and 2 in "abcd" results in "cbad".
Example 1:
Input: A = "ab", B = "ba"
Output: true
Explanation: You can swap A[0] = 'a' and A[1] = 'b' to get "ba", which is equal to B.
Example 2:
Input: A = "ab", B = "ab"
Output: false
Explanation: The only letters you can swap are A[0] = 'a' and A[1] = 'b', which results in "ba" != B.
Example 3:
Input: A = "aa", B = "aa"
Output: true
Explanation: You can swap A[0] = 'a' and A[1] = 'a' to get "aa", which is equal to B.
Example 4:
Input: A = "aaaaaaabc", B = "aaaaaaacb"
Output: true
Example 5:
Input: A = "", B = "aa"
Output: false
Constraints:
- 0 <= A.length <= 20000
- 0 <= B.length <= 20000
- A and B consist of lowercase letters.
Solution
class Solution:
def buddyStrings(self, A: str, B: str) -> bool:
if len(A) != len(B):
return False
else:
list_A = list(A)
list_B = list(B)
check_string_A = []
check_string_B = []
for i in range(len(list_A)):
if list_A[i] != list_B[i]:
check_string_A.append(list_A[i])
check_string_B.append(list_B[i])
if len(check_string_A) > 2:
return False
if len(check_string_A) == 2 and check_string_A[::-1] == check_string_B:
return True
if len(check_string_A) == 0:
if len(list_A) > len(set(list_A)):
return True
return False
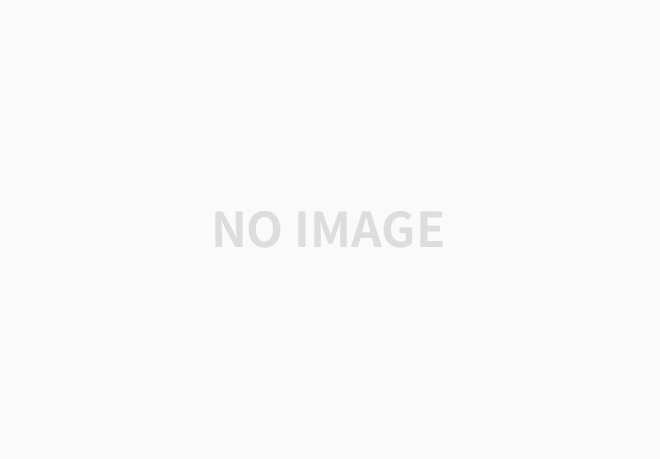
SOMJANG/CODINGTEST_PRACTICE
1일 1문제 since 2020.02.07. Contribute to SOMJANG/CODINGTEST_PRACTICE development by creating an account on GitHub.
github.com
'Programming > 코딩 1일 1문제' 카테고리의 다른 글
[leetCode] 397. Integer Replacement (Python) (0) | 2020.11.15 |
---|---|
[leetCode] 747. Largest Number At Least Twice of Others (Python) (0) | 2020.11.13 |
[leetCode] 1394. Find Lucky Integer in an Array (Python) (0) | 2020.11.11 |
[leetCode] 81. Search in Rotated Sorted Array II (Python) (0) | 2020.11.10 |
[leetCode] 867. Transpose Matrix (Python) (0) | 2020.11.09 |