Notice
Recent Posts
Recent Comments
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- ubuntu
- 파이썬
- 자연어처리
- Real or Not? NLP with Disaster Tweets
- gs25
- hackerrank
- leetcode
- Git
- 프로그래머스 파이썬
- dacon
- ChatGPT
- 편스토랑
- Docker
- 데이콘
- 백준
- 우분투
- Kaggle
- 캐치카페
- PYTHON
- AI 경진대회
- github
- 코로나19
- 더현대서울 맛집
- 프로그래머스
- 맥북
- programmers
- Baekjoon
- 편스토랑 우승상품
- 금융문자분석경진대회
- SW Expert Academy
Archives
- Today
- Total
솜씨좋은장씨
[leetCode] 443. String Compression (Python) 본문
728x90
반응형
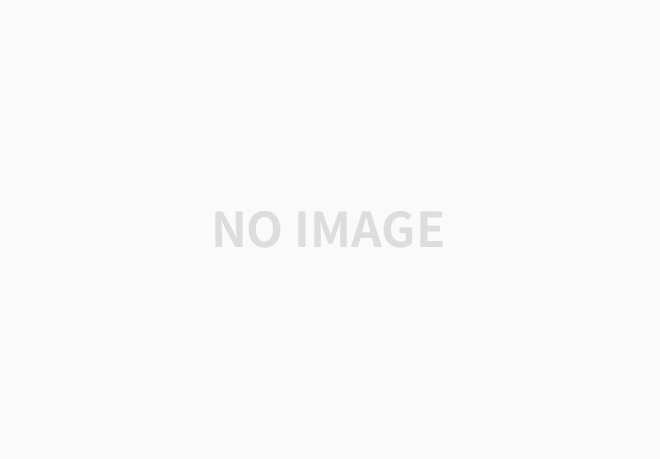
Given an array of characters, compress it in-place.
The length after compression must always be smaller than or equal to the original array.
Every element of the array should be a character (not int) of length 1.
After you are done modifying the input array in-place, return the new length of the array.
Follow up:
Could you solve it using only O(1) extra space?
Example 1:
Input:
["a","a","b","b","c","c","c"]
Output:
Return 6, and the first 6 characters of the input array should be: ["a","2","b","2","c","3"]
Explanation:
"aa" is replaced by "a2". "bb" is replaced by "b2". "ccc" is replaced by "c3".
Example 2:
Input:
["a"]
Output:
Return 1, and the first 1 characters of the input array should be: ["a"]
Explanation:
Nothing is replaced.
Example 3:
Input:
["a","b","b","b","b","b","b","b","b","b","b","b","b"]
Output:
Return 4, and the first 4 characters of the input array should be: ["a","b","1","2"].
Explanation:
Since the character "a" does not repeat, it is not compressed. "bbbbbbbbbbbb" is replaced by "b12".
Notice each digit has it's own entry in the array.
Note:
- All characters have an ASCII value in [35, 126].
- 1 <= len(chars) <= 1000.
Solution
class Solution:
def compress(self, chars: List[str]) -> int:
stack = []
nums = 1
idx = 0
if len(chars) > 0:
stack.append(chars[0])
for i in range(1, len(chars)):
if chars[i] == stack[idx]:
nums = nums + 1
if i == len(chars) - 1:
num_list = list(str(nums))
idx = idx + 1 + len(num_list)
stack = stack + num_list
elif chars[i] != stack[idx]:
if nums == 1:
idx = idx + 1
num_list = list(str(nums))
if num_list != ['1']:
idx = idx + 1 + len(num_list)
stack = stack + num_list
stack.append(chars[i])
nums = 1
for i in range(len(stack)):
chars[i] = stack[i]
chars = chars[0:len(stack)]
return len(chars)
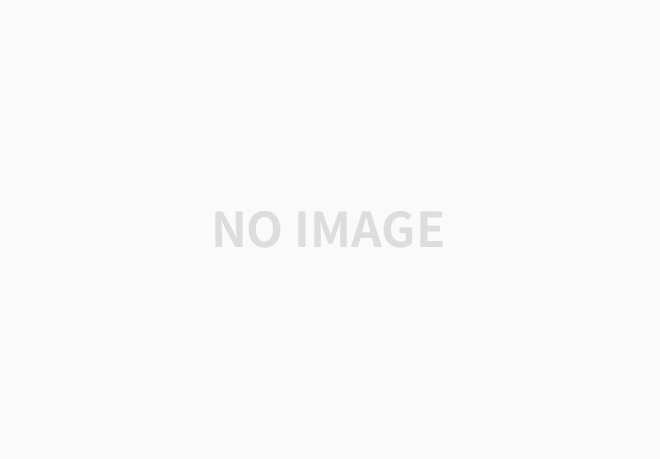
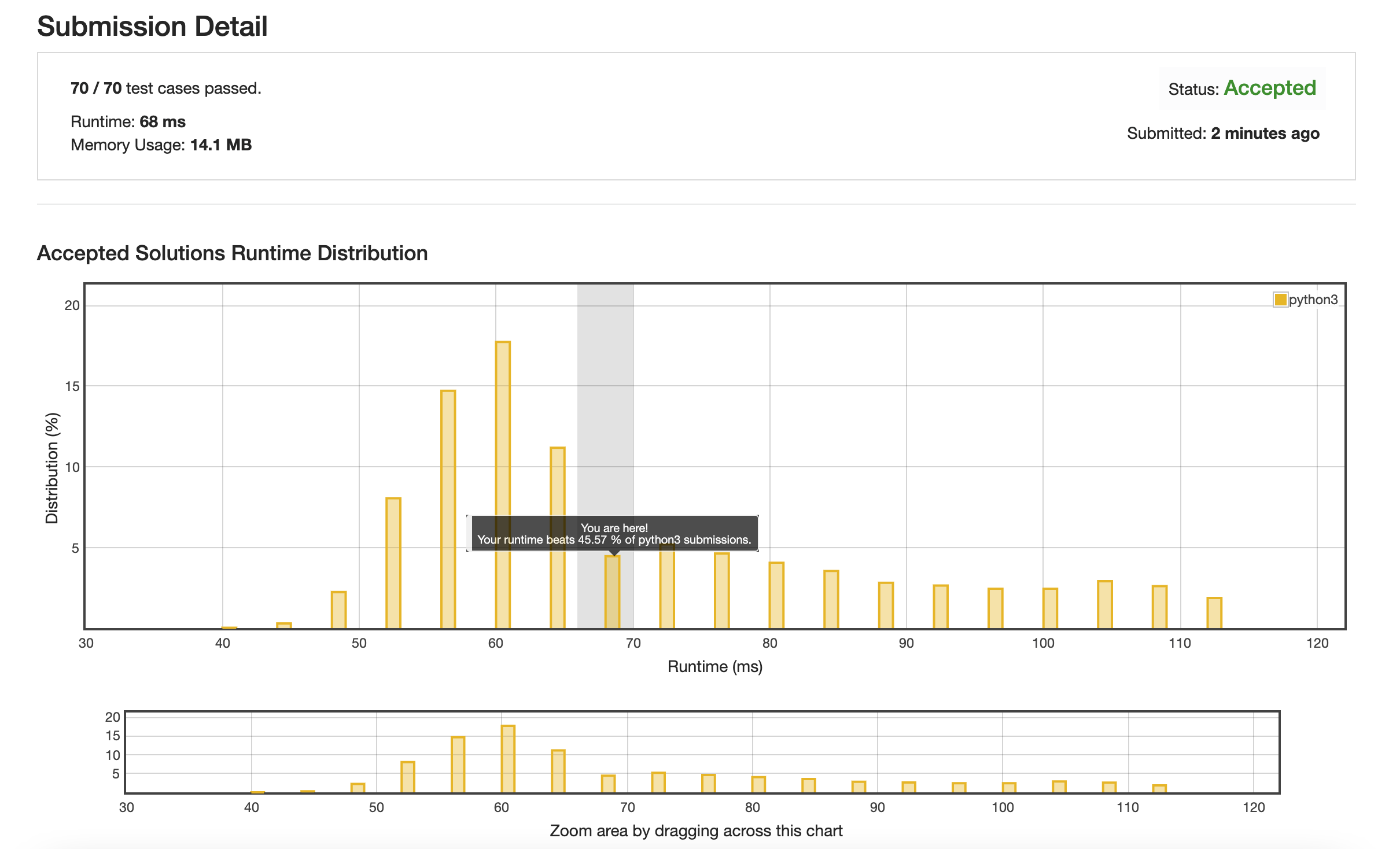
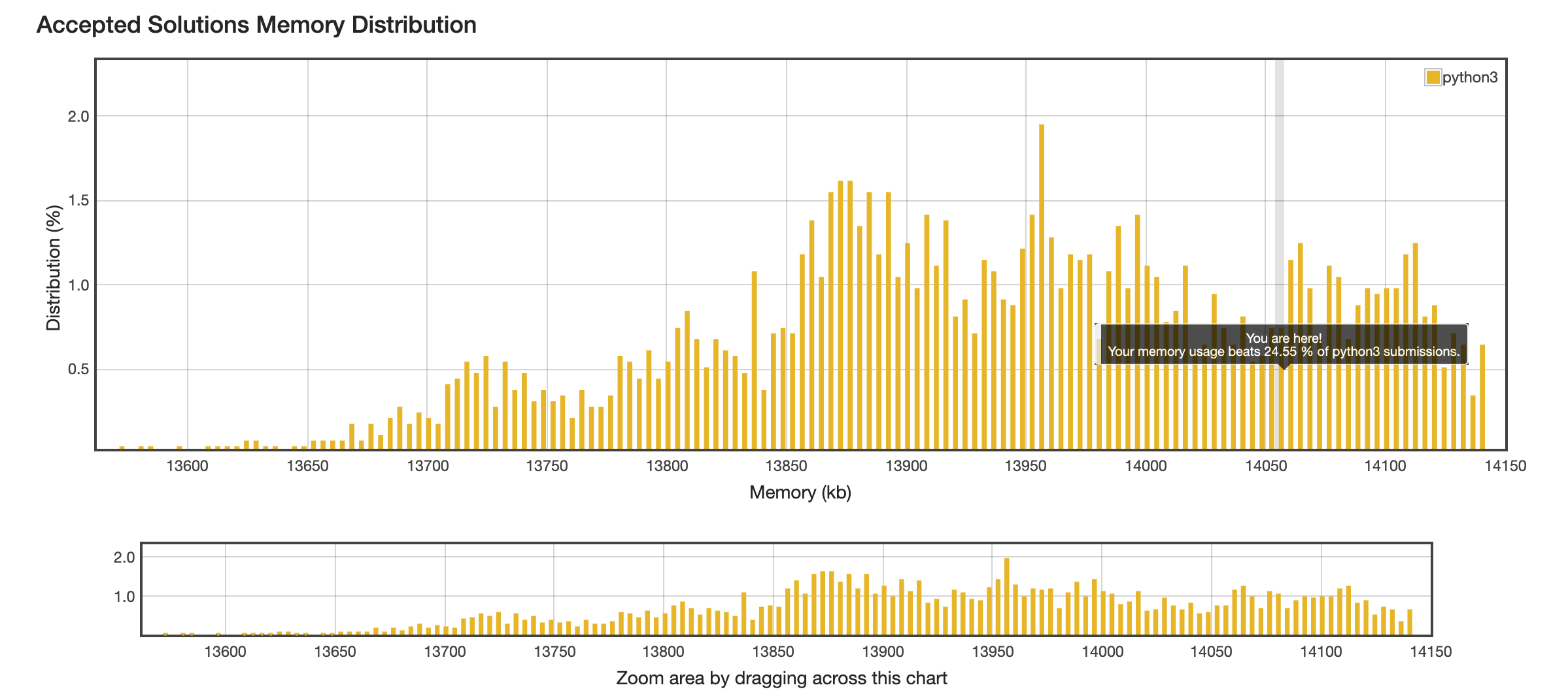
SOMJANG/CODINGTEST_PRACTICE
1일 1문제 since 2020.02.07. Contribute to SOMJANG/CODINGTEST_PRACTICE development by creating an account on GitHub.
github.com
'Programming > 코딩 1일 1문제' 카테고리의 다른 글
[leetCode] 415. Add Strings (Python) (0) | 2020.09.05 |
---|---|
[leetCode] 917. Reverse Only Letters (Python) (0) | 2020.09.04 |
[leetCode] 1480. Running Sum of 1d Array (Python) (0) | 2020.09.02 |
[leetCode] 728. Self Dividing Numbers (Python) (0) | 2020.09.01 |
[leetCode] 977. Squares of a Sorted Array (Python) (0) | 2020.09.01 |