Notice
Recent Posts
Recent Comments
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- Real or Not? NLP with Disaster Tweets
- ubuntu
- leetcode
- Git
- SW Expert Academy
- Baekjoon
- 캐치카페
- Kaggle
- 금융문자분석경진대회
- github
- 백준
- 자연어처리
- 코로나19
- ChatGPT
- Docker
- programmers
- dacon
- 데이콘
- 편스토랑
- 프로그래머스 파이썬
- 더현대서울 맛집
- PYTHON
- 프로그래머스
- gs25
- 우분투
- hackerrank
- 맥북
- 파이썬
- 편스토랑 우승상품
- AI 경진대회
Archives
- Today
- Total
솜씨좋은장씨
[leetCode] 17. Letter Combinations of a Phone Number (Python) 본문
Programming/코딩 1일 1문제
[leetCode] 17. Letter Combinations of a Phone Number (Python)
솜씨좋은장씨 2020. 10. 6. 19:08728x90
반응형
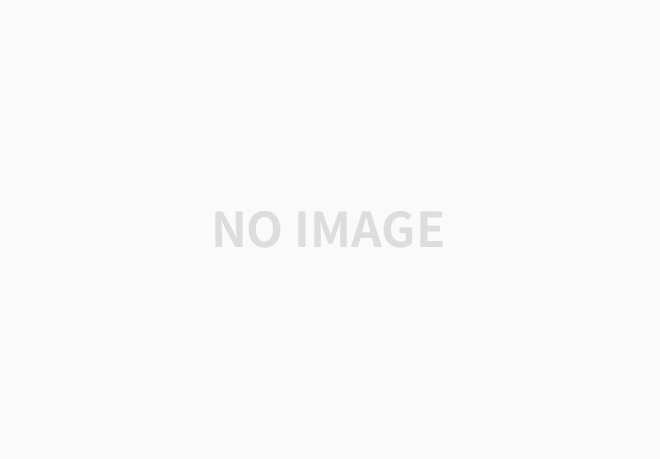
Given a string containing digits from 2-9 inclusive, return all possible letter combinations that the number could represent. Return the answer in any order.
A mapping of digit to letters (just like on the telephone buttons) is given below. Note that 1 does not map to any letters.
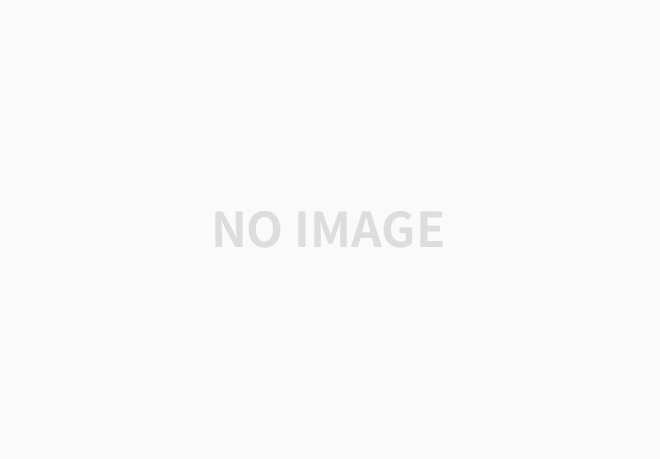
Example 1:
Input: digits = "23"
Output: ["ad","ae","af","bd","be","bf","cd","ce","cf"]
Example 2:
Input: digits = ""
Output: []
Example 3:
Input: digits = "2"
Output: ["a","b","c"]
Constraints:
- 0 <= digits.length <= 4
- digits[i] is a digit in the range ['2', '9'].
Solution
from itertools import combinations
class Solution:
def letterCombinations(self, digits: str) -> List[str]:
num2word = {"2":['a', 'b', 'c'], "3":['d', 'e', 'f'], "4":['g', 'h', 'i'], "5":['j', 'k', 'l'],
"6":['m', 'n', 'o'], "7":['p', 'q', 'r', 's'], "8":['t', 'u', 'v'], "9":['w', 'x', 'y', 'z']}
if digits == "":
return []
else:
digit_num_list = list(digits)
if len(digit_num_list) == 1:
return num2word[digit_num_list[0]]
else:
word_list = [num2word[digit_num] for digit_num in digit_num_list]
pre_list = word_list[0]
post_lists = word_list[1:]
# print("pre_list : {}, post_lists : {}".format(pre_list, post_lists))
for post_list in post_lists:
comb_list = self.make_combination_list(pre_list, post_list)
pre_list = comb_list
# print("result : {}".format(comb_list))
return comb_list
@classmethod
def make_combination_list(self, list1, list2):
my_comb = []
# print("list1 : {}, list2: {}".format(list1, list2))
for word1 in list1:
for word2 in list2:
my_comb.append(word1 + word2)
return my_comb
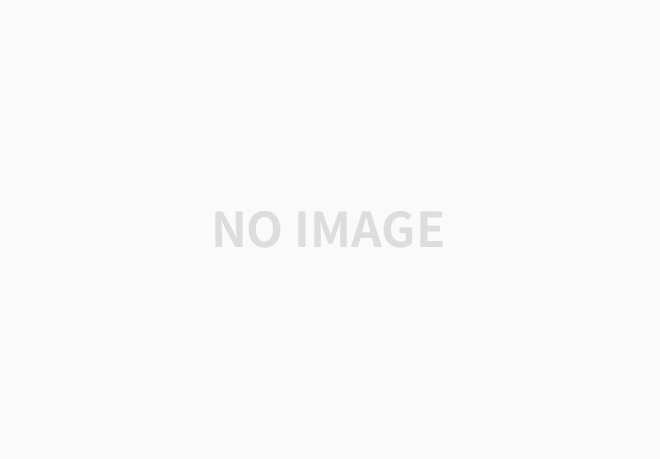
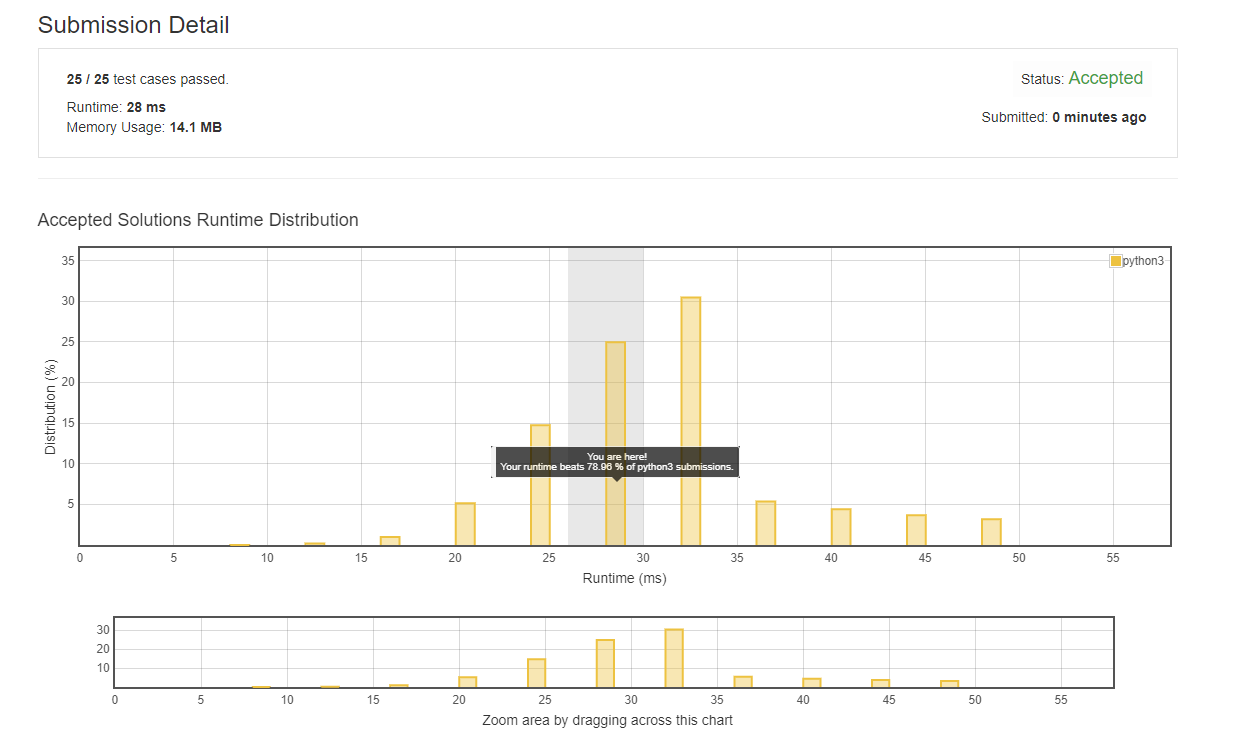
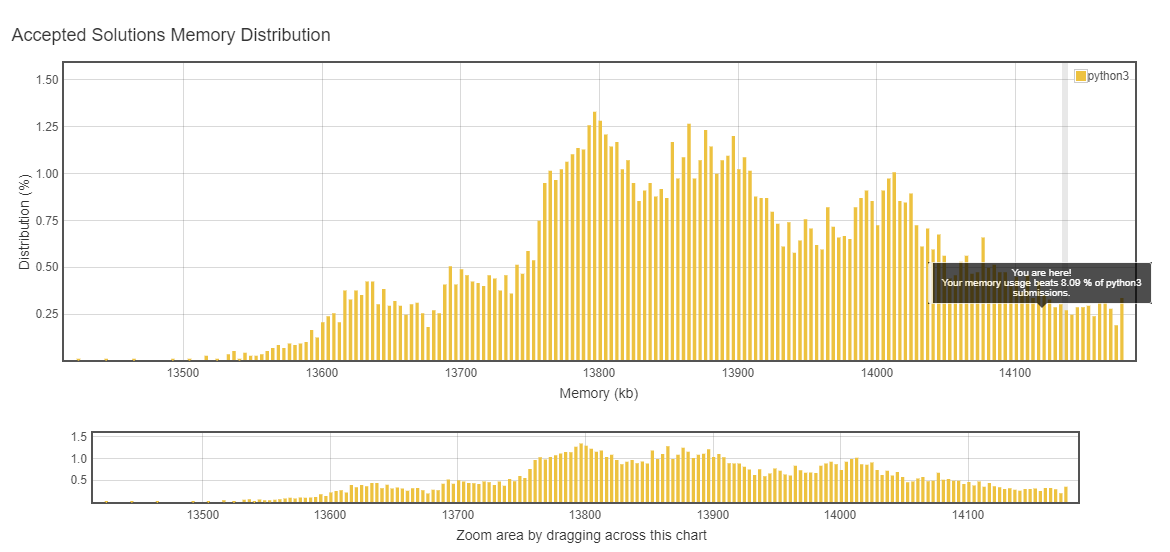
SOMJANG/CODINGTEST_PRACTICE
1일 1문제 since 2020.02.07. Contribute to SOMJANG/CODINGTEST_PRACTICE development by creating an account on GitHub.
github.com
'Programming > 코딩 1일 1문제' 카테고리의 다른 글
[leetCode] 258. Add Digits (Python) (0) | 2020.10.08 |
---|---|
[leetCode] 1089. Duplicate Zeros (Python) (0) | 2020.10.07 |
[leetCode] 350. Intersection of Two Arrays II (Python) (0) | 2020.10.05 |
[leetCode] 729. My Calendar I (Python) (2) | 2020.10.04 |
[leetCode] 1492. The kth Factor of n (Python) (0) | 2020.10.03 |