일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- hackerrank
- gs25
- 캐치카페
- github
- PYTHON
- 더현대서울 맛집
- AI 경진대회
- ubuntu
- 프로그래머스
- 자연어처리
- 프로그래머스 파이썬
- 맥북
- Real or Not? NLP with Disaster Tweets
- Kaggle
- 편스토랑
- SW Expert Academy
- 우분투
- 코로나19
- 금융문자분석경진대회
- 파이썬
- 데이콘
- Docker
- 백준
- 편스토랑 우승상품
- Git
- programmers
- ChatGPT
- Baekjoon
- dacon
- leetcode
- Today
- Total
솜씨좋은장씨
[leetCode] 165. Compare Version Numbers (Python) 본문
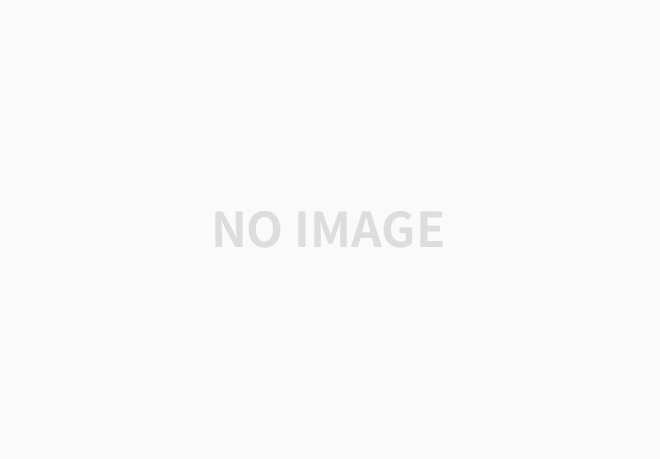
Given two version numbers, version1 and version2, compare them.
Version numbers consist of one or more revisions joined by a dot '.'. Each revision consists of digits and may contain leading zeros. Every revision contains at least one character. Revisions are 0-indexed from left to right, with the leftmost revision being revision 0, the next revision being revision 1, and so on. For example 2.5.33 and 0.1 are valid version numbers.
To compare version numbers, compare their revisions in left-to-right order. Revisions are compared using their integer value ignoring any leading zeros. This means that revisions 1 and 001 are considered equal. If a version number does not specify a revision at an index, then treat the revision as 0. For example, version 1.0 is less than version 1.1 because their revision 0s are the same, but their revision 1s are 0 and 1 respectively, and 0 < 1.
Return the following:
- If version1 < version2, return -1.
- If version1 > version2, return 1.
- Otherwise, return 0.
Example 1:
Input: version1 = "1.01", version2 = "1.001"
Output: 0
Explanation: Ignoring leading zeroes, both "01" and "001" represent the same integer "1".
Example 2:
Input: version1 = "1.0", version2 = "1.0.0"
Output: 0
Explanation: version1 does not specify revision 2, which means it is treated as "0".
Example 3:
Input: version1 = "0.1", version2 = "1.1"
Output: -1
Explanation: version1's revision 0 is "0", while version2's revision 0 is "1". 0 < 1, so version1 < version2.
Example 4:
Input: version1 = "1.0.1", version2 = "1"
Output: 1
Example 5:
Input: version1 = "7.5.2.4", version2 = "7.5.3"
Output: -1
Constraints:
- 1 <= version1.length, version2.length <= 500
- version1 and version2 only contain digits and '.'.
- version1 and version2 are valid version numbers.
- All the given revisions in version1 and version2 can be stored in a 32-bit integer.
Solution
class Solution:
def compareVersion(self, version1: str, version2: str) -> int:
version1_split = list(map(int, version1.split(".")))
version2_split = list(map(int, version2.split(".")))
max_len = max(len(version1_split), len(version2_split))
if len(version1_split) != len(version2_split):
if len(version1_split) == max_len:
version2_split = version2_split + [0] * (max_len - len(version2_split))
elif len(version2_split) == max_len:
version1_split = version1_split + [0] * (max_len - len(version1_split))
for i in range(max_len):
if version1_split[i] > version2_split[i]:
return 1
elif version1_split[i] < version2_split[i]:
return -1
return 0
Solution 해설
먼저 각 자리수로 비교하기 위해서 1.0.0 에서 . 을 기준으로 split 해줍니다.
그 다음 어떻게 할 까 하다가 pad_sequences 가 떠올랐고 짧은 길이의 리스트의 길이를 긴 리스트랑 맞춰주기로 했습니다.
그 다음 0번째 Index 부터 비교하면서 version1 리스트의 숫자가 더 크면 1 작으면 -1 만약 모든 반복문을 완료하면 0을
return 하도록 합니다.
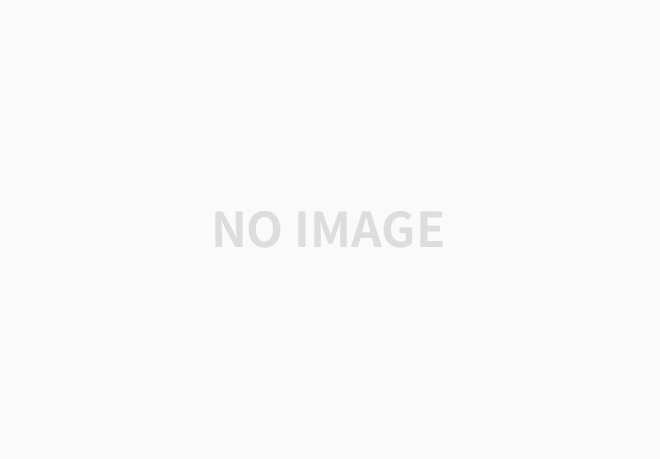
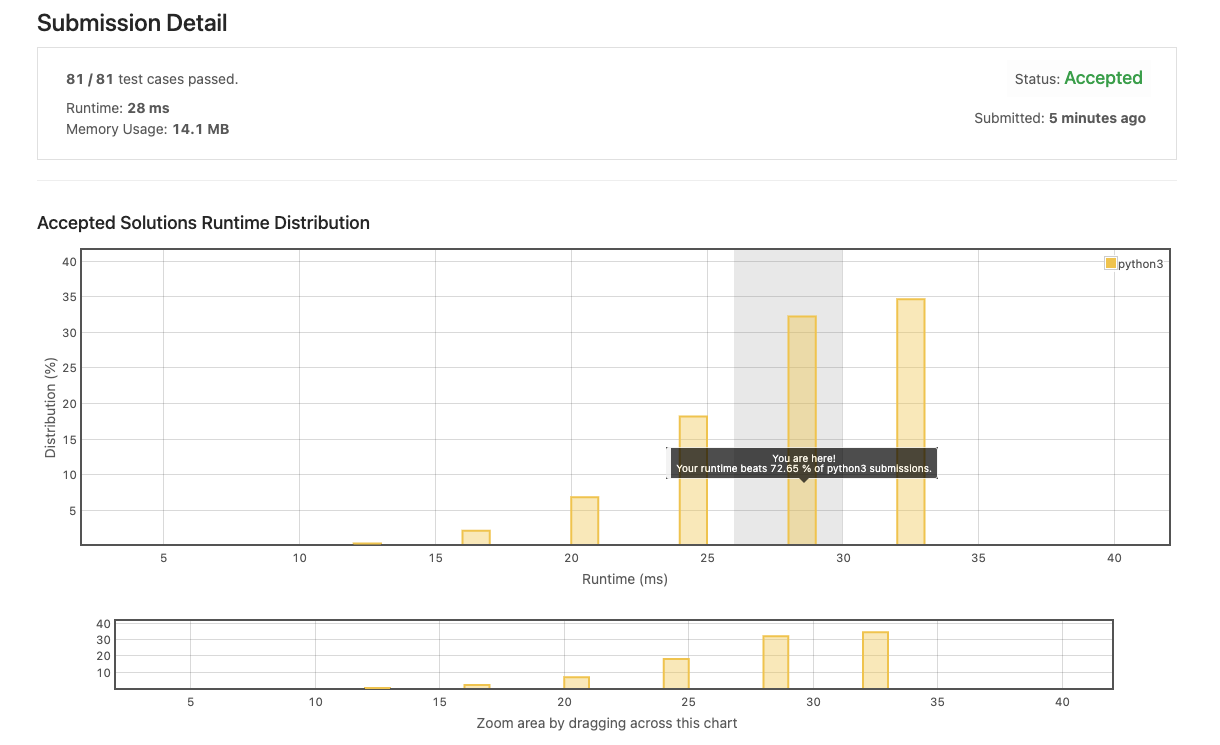
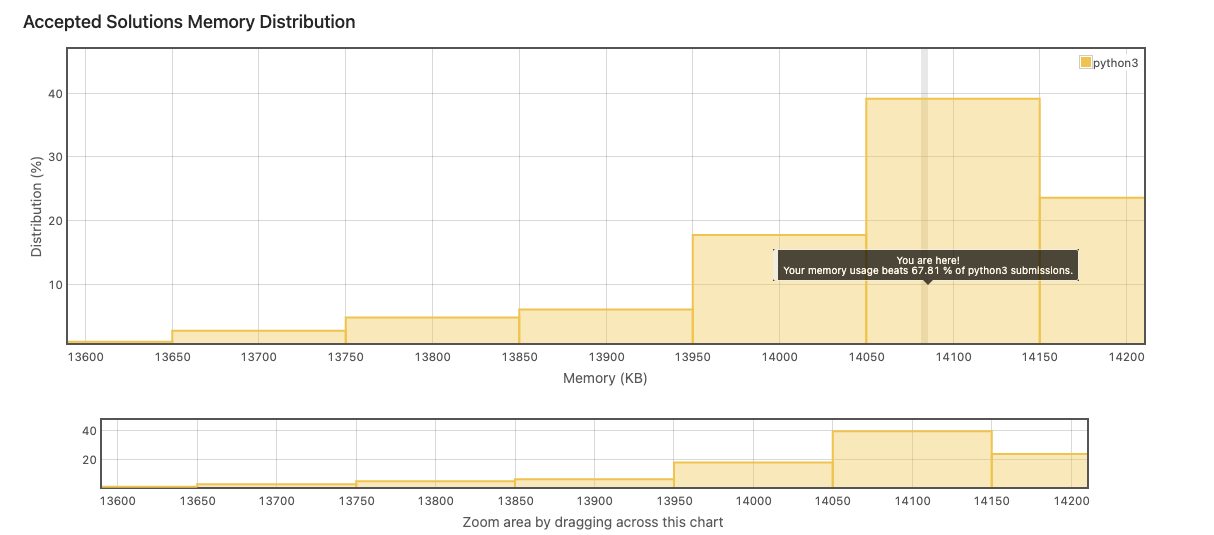
SOMJANG/CODINGTEST_PRACTICE
1일 1문제 since 2020.02.07. Contribute to SOMJANG/CODINGTEST_PRACTICE development by creating an account on GitHub.
github.com
'Programming > 코딩 1일 1문제' 카테고리의 다른 글
[leetCode] 338. Counting Bits (Pyhton) (0) | 2020.11.21 |
---|---|
[leetCode] 313. Super Ugly Number (Python) (0) | 2020.11.20 |
[leetCode] 77. Combinations (Python) (0) | 2020.11.18 |
[leetCode] 47. Permutations II (Python) (0) | 2020.11.17 |
[leetCode] 19. Remove Nth Node From End of List (Python) (0) | 2020.11.16 |