일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- programmers
- 편스토랑
- Real or Not? NLP with Disaster Tweets
- hackerrank
- 코로나19
- leetcode
- 자연어처리
- 우분투
- dacon
- Baekjoon
- ubuntu
- Git
- 더현대서울 맛집
- Kaggle
- gs25
- Docker
- ChatGPT
- 맥북
- 편스토랑 우승상품
- 파이썬
- SW Expert Academy
- AI 경진대회
- PYTHON
- 프로그래머스
- 캐치카페
- 데이콘
- 금융문자분석경진대회
- 프로그래머스 파이썬
- github
- 백준
- Today
- Total
솜씨좋은장씨
[Python] Pycharm에서 unittest 사용해보기! 본문
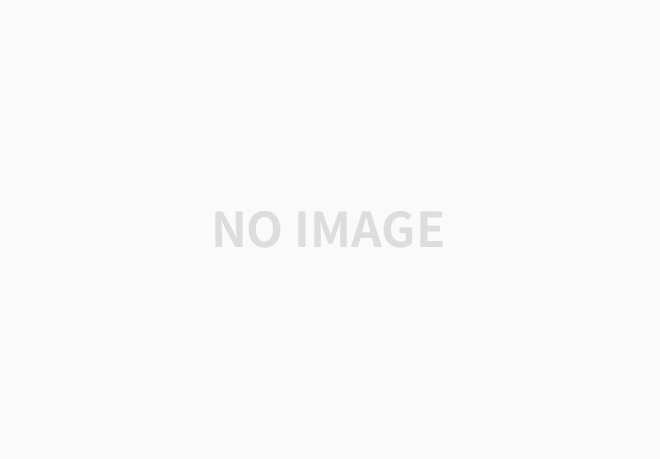
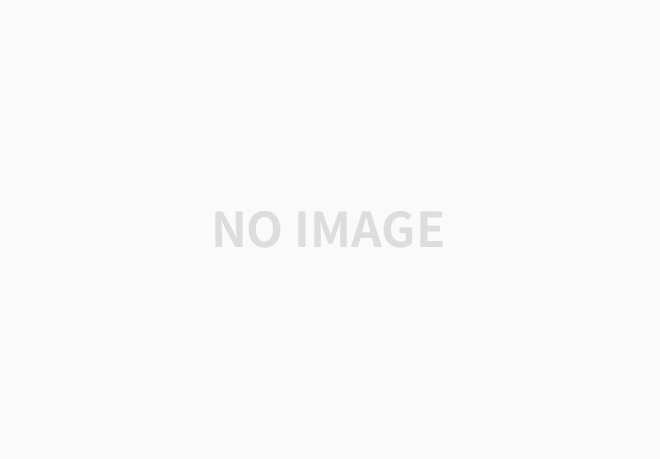
Pycharm에서 unittest를 사용하는 방법에 대해서 정리해보려합니다.
Step 3. Test your first Python application - Help | PyCharm
Step 3. Test your first Python application Remember, in the first tutorial you’ve created your first Python application, and in the second tutorial you’ve debugged it. Now it’s time to do some testing. Choosing the test runner If you used nosetest, py.test
www.jetbrains.com
위의 문서를 참조하여 작성하였습니다.
1. 테스트에 사용할 프로젝트 만들기
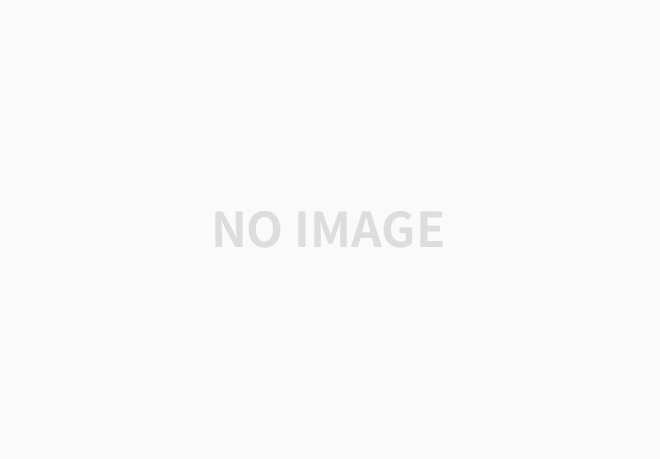
테스트에 사용할 프로젝트 하나를 생성하였습니다.
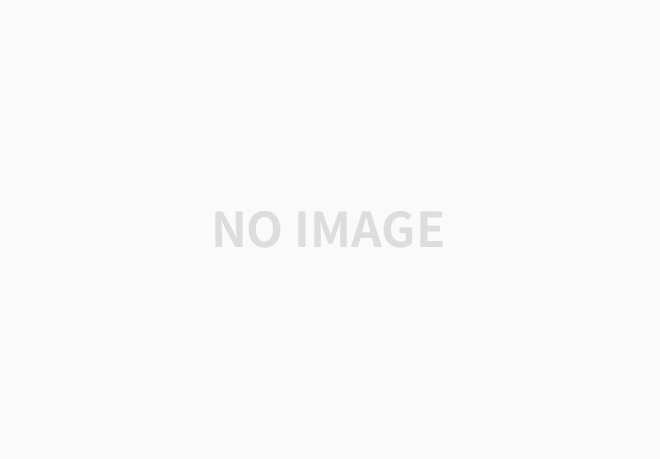
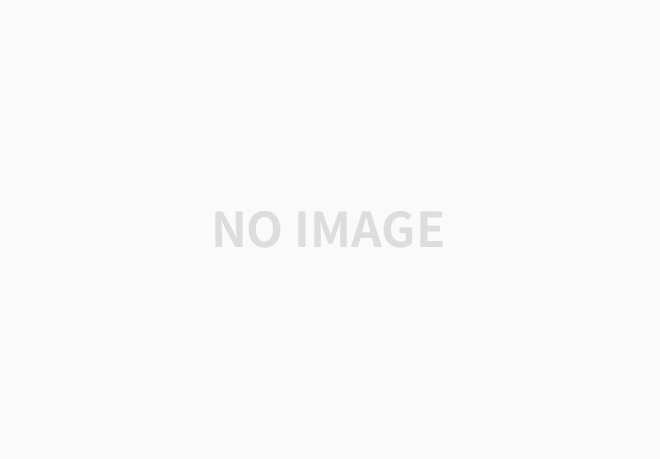
프로젝트가 생성되었다면 Car 이라는 파일을 하나 생성합니다.
Car.py
class Car:
def __init__(self, speed=0):
self.speed = speed
self.odometer = 0
self.time = 0
def say_state(self):
print("현재 차량의 속도는 {} Kph 입니다.".format(self.speed))
def accelerate(self):
self.speed += 5
def brake(self):
self.speed -= 5
def step(self):
self.odometer += self.speed
self.time += 1
def average_speed(self):
if self.time != 0:
return self.odometer / self.time
else:
pass
if __name__ == '__main__':
my_car = Car()
print("차량에 연결되었습니다.")
while True:
action = input("[A] 가속 [B] 감속 [O] 주행거리 [S] 평균속도")
if action not in "ABOS" or len(action) != 1:
print("해당 명령은 실행할 수 없습니다.")
continue
if action == 'A':
my_car.accelerate()
elif action == 'B':
my_car.brake()
elif action == 'O':
print("현재까지 주행거리는 {} km 입니다.".format(my_car.odometer))
elif action =='S':
print("평균속도는 {} kph 입니다.".format(my_car.average_speed()))
my_car.step()
my_car.say_state()
다음의 내용을 작성합니다.
2. Test runner 선택하기
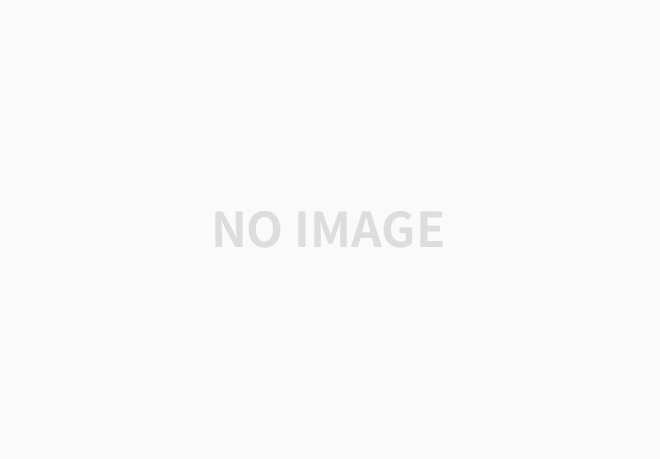
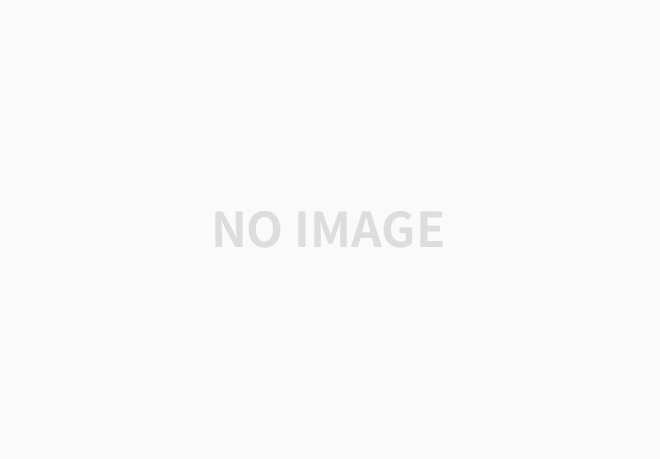
Preference > Tools > Python Integrated Tools > Testing > Default test runner : Unittests 를 선택합니다.
3. Test 생성하기
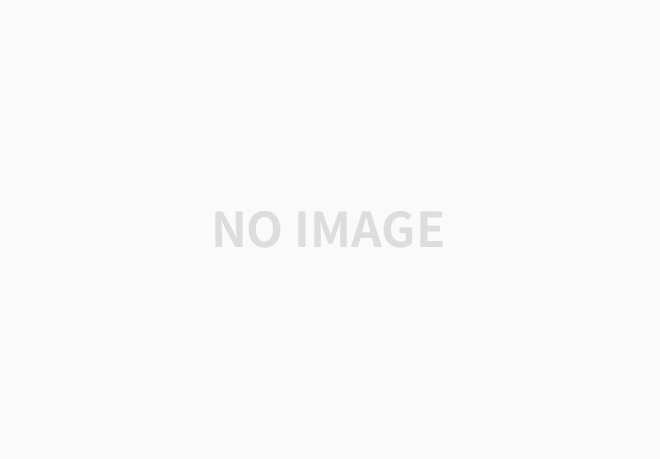
Car.py의 코드창에 오른쪽 마우스를 클릭하여 나오는 메뉴에서
Go To > Test 를 클릭합니다.
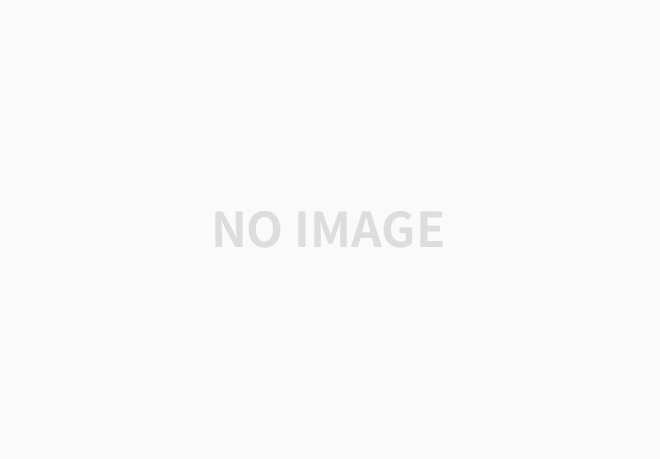
그럼 나오는 Create New Test를 클릭합니다.
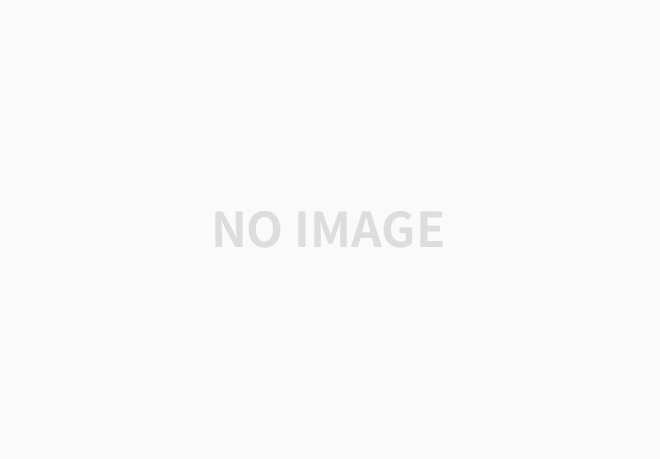
테스트를 하고자하는 항목을 선택합니다.
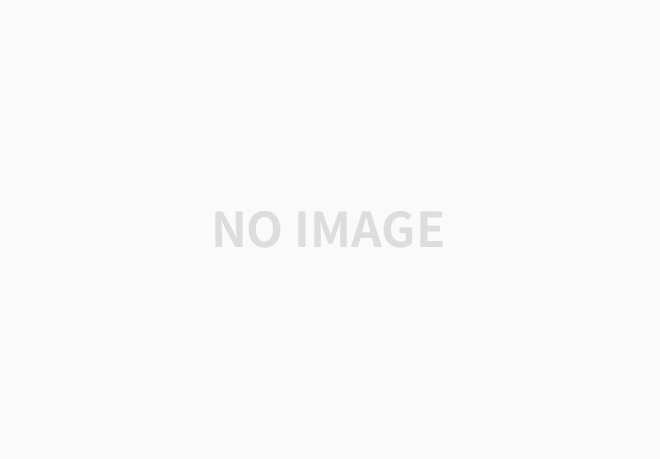
그럼 자동으로 test_car.py 가 생성되는 것을 확인할 수 있습니다.
4. Test 실행해보기
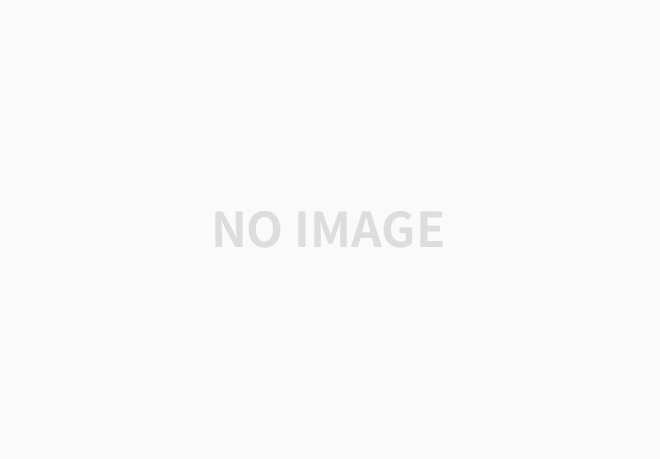
class TestCar(TestCase):
코드 옆에있는 화살표를 눌러 테스트를 실행해봅니다.
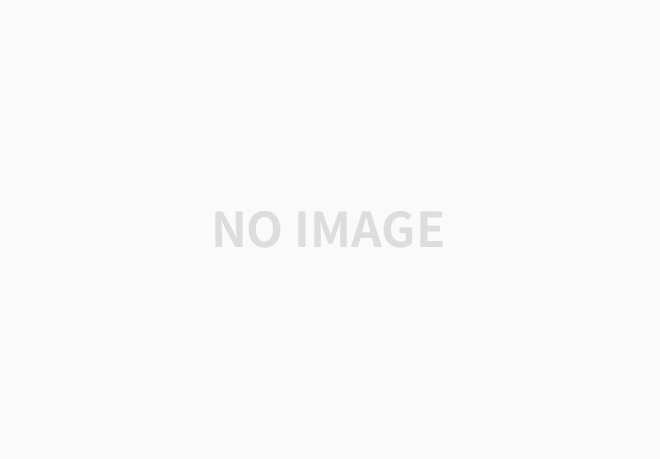
테스트가 제대로 실행되지 않은 것을 볼 수 있습니다.
아직 안에 아무것도 없기 때문입니다.
test_car.py
import unittest
from Car import Car
class TestCar(unittest.TestCase):
def setUp(self):
self.car = Car()
class TestInit(TestCar):
def test_initial_speed(self):
self.assertEqual(self.car.speed, 0)
def test_initial_odometer(self):
self.assertEqual(self.car.odometer, 0)
def test_initial_time(self):
self.assertEqual(self.car.time, 0)
class TestAccelerate(TestCar):
def test_accelerate_from_zero(self):
self.car.accelerate()
self.assertEqual(self.car.speed, 5)
def test_multiple_accelerates(self):
for _ in range(3):
self.car.accelerate()
self.assertEqual(self.car.speed, 15)
class TestBrake(TestCar):
def test_brake_once(self):
self.car.accelerate()
self.car.brake()
self.assertEqual(self.car.speed, 0)
def test_multiple_brakes(self):
for _ in range(5):
self.car.accelerate()
for _ in range(3):
self.car.brake()
self.assertEqual(self.car.speed, 10)
def test_should_not_allow_negative_speed(self):
self.car.brake()
self.assertEqual(self.car.speed, 0)
def test_multiple_brakes_at_zero(self):
for _ in range(3):
self.car.brake()
self.assertEqual(self.car.speed, 0)
코드를 수정하고 다시 실행해보았습니다.
이때 아까와 같이 화살표를 눌러 실행하게 되면
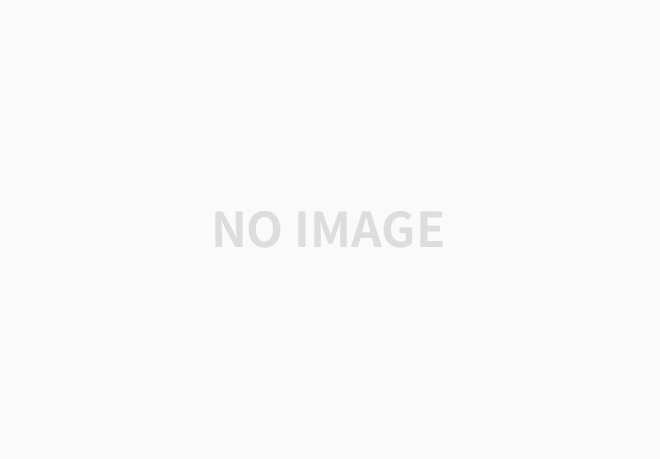
No tests were found
라는 에러를 보게 됩니다.
이 No tests were found 오류는 class 안에 함수의 이름이 test_로 시작하지 않아 발생하는 오류입니다.
여기서는 class TestCar(unittest.TestCase) 안에 setUp(self) 때문인데
이는 화살표를 클릭하여 실행하는 방법이 아닌 코드창에서 오른쪽 마우스 클릭을 통해 실행하면
No tests were found 에러없이 테스트를 수행합니다.
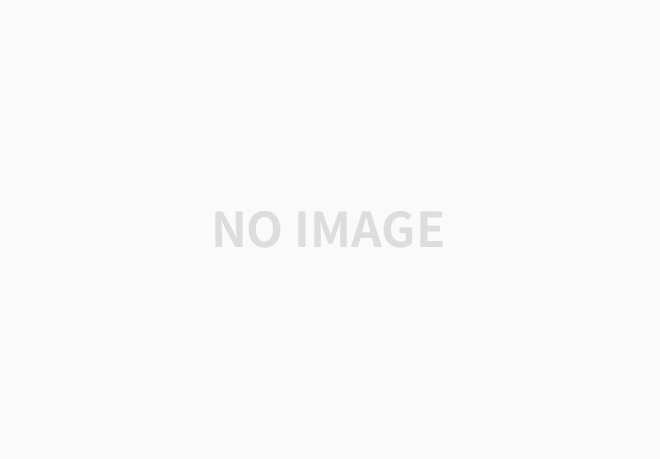
결과
Testing started at 5:53 오후 ...
/Users/donghyunjang/PycharmProjects/MyTestPractice/venv/bin/python "/Applications/PyCharm CE.app/Contents/helpers/pycharm/_jb_unittest_runner.py" --path /Users/donghyunjang/PycharmProjects/MyTestPractice/test_car.py
Launching unittests with arguments python -m unittest /Users/donghyunjang/PycharmProjects/MyTestPractice/test_car.py in /Users/donghyunjang/PycharmProjects/MyTestPractice
0 != -15
Expected :-15
Actual :0
<Click to see difference>
Traceback (most recent call last):
File "/Applications/PyCharm CE.app/Contents/helpers/pycharm/teamcity/diff_tools.py", line 32, in _patched_equals
old(self, first, second, msg)
File "/Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/unittest/case.py", line 839, in assertEqual
assertion_func(first, second, msg=msg)
File "/Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/unittest/case.py", line 832, in _baseAssertEqual
raise self.failureException(msg)
AssertionError: -15 != 0
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/unittest/case.py", line 59, in testPartExecutor
yield
File "/Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/unittest/case.py", line 615, in run
testMethod()
File "/Users/donghyunjang/PycharmProjects/MyTestPractice/test_car.py", line 52, in test_multiple_brakes_at_zero
self.assertEqual(self.car.speed, 0)
Ran 9 tests in 0.034s
FAILED (failures=2)
0 != -5
Expected :-5
Actual :0
<Click to see difference>
Traceback (most recent call last):
File "/Applications/PyCharm CE.app/Contents/helpers/pycharm/teamcity/diff_tools.py", line 32, in _patched_equals
old(self, first, second, msg)
File "/Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/unittest/case.py", line 839, in assertEqual
assertion_func(first, second, msg=msg)
File "/Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/unittest/case.py", line 832, in _baseAssertEqual
raise self.failureException(msg)
AssertionError: -5 != 0
During handling of the above exception, another exception occurred:
Traceback (most recent call last):
File "/Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/unittest/case.py", line 59, in testPartExecutor
yield
File "/Library/Frameworks/Python.framework/Versions/3.7/lib/python3.7/unittest/case.py", line 615, in run
testMethod()
File "/Users/donghyunjang/PycharmProjects/MyTestPractice/test_car.py", line 47, in test_should_not_allow_negative_speed
self.assertEqual(self.car.speed, 0)
Process finished with exit code 1
Assertion failed
Assertion failed
Assertion failed
테스트를 잘 수행은 하였으나
9개의 테스트 중 7개는 패스하였으나 2개는 실패한 것을 볼 수 있습니다.
다음은 이 테스트 결과를 토대로 디버깅을 해보려합니다.
읽어주셔서 감사합니다.
'Programming > Python' 카테고리의 다른 글
[Python] Python3에서 venv로 가상환경 만들고 사용하기! (0) | 2020.04.06 |
---|---|
[Python] Pycharm에서 디버깅하기! (0) | 2020.04.04 |
[Python] Sphinx 를 활용하여 Python 문서화해보기! (0) | 2020.04.02 |
[Python] List Comprehension과 리스트를 다루는 여러가지 방법들! (0) | 2020.04.02 |
[Python] 2to3 를 통해 Python 2로 작성된 코드를 Python3로 쉽게 바꾸어보기! (2) | 2020.04.02 |