일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- 금융문자분석경진대회
- programmers
- 캐치카페
- 백준
- 자연어처리
- hackerrank
- 파이썬
- SW Expert Academy
- gs25
- 편스토랑
- Baekjoon
- github
- Docker
- leetcode
- AI 경진대회
- Git
- dacon
- 코로나19
- Real or Not? NLP with Disaster Tweets
- 편스토랑 우승상품
- 우분투
- 더현대서울 맛집
- ubuntu
- 데이콘
- 프로그래머스
- 프로그래머스 파이썬
- 맥북
- ChatGPT
- PYTHON
- Kaggle
- Today
- Total
솜씨좋은장씨
[HackerRank] Stacks and Queues : Balanced Brackets (Python) 본문
[HackerRank] Stacks and Queues : Balanced Brackets (Python)
솜씨좋은장씨 2020. 3. 20. 12:27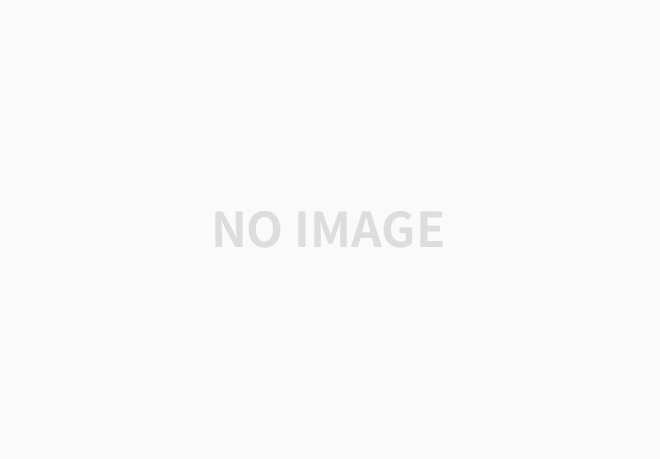
A bracket is considered to be any one of the following characters: (, ), {, }, [, or ].
Two brackets are considered to be a matched pair if the an opening bracket (i.e., (, [, or {) occurs to the left of a closing bracket (i.e., ), ], or }) of the exact same type. There are three types of matched pairs of brackets: [], {}, and ().
A matching pair of brackets is not balanced if the set of brackets it encloses are not matched. For example, {[(])} is not balanced because the contents in between { and } are not balanced. The pair of square brackets encloses a single, unbalanced opening bracket, (, and the pair of parentheses encloses a single, unbalanced closing square bracket, ].
By this logic, we say a sequence of brackets is balanced if the following conditions are met:
- It contains no unmatched brackets.
- The subset of brackets enclosed within the confines of a matched pair of brackets is also a matched pair of brackets.
Given n strings of brackets, determine whether each sequence of brackets is balanced. If a string is balanced, return YES. Otherwise, return NO.
Function Description
Complete the function isBalanced in the editor below. It must return a string: YES if the sequence is balanced or NO if it is not.
isBalanced has the following parameter(s):
- s: a string of brackets
Input Format
The first line contains a single integer n, the number of strings.
Each of the next n lines contains a single string s, a sequence of brackets.
Constraints
- 1 <= n <= 10^3
- 1<= | s | <= 10^3, where | s | is the length of the sequence.
- All chracters in the sequences ∈ { {, }, (, ), [, ] }.
Output Format
For each string, return YES or NO.
Sample Input
3
{[()]}
{[(])}
{{[[(())]]}}
Sample Output
YES
NO
YES
Explanation
- The string {[()]} meets both criteria for being a balanced string, so we print YES on a new line.
- The string {[(])} is not balanced because the brackets enclosed by the matched pair { and } are not balanced: [(]).
- The string {{[[(())]]}} meets both criteria for being a balanced string, so we print YES on a new line.
첫번째 코드
#!/bin/python3
import math
import os
import random
import re
import sys
# Complete the isBalanced function below.
def isBalanced(s):
front = 0
end = len(s) -1
answer = "YES"
for i in range(len(s) // 2):
if s[front + i] == '{':
if s[end-i] != '}':
answer = "NO"
break
elif s[front + i] == '(':
if s[end-i] != ')':
answer = "NO"
break
elif s[front + i] == '[':
if s[end-i] != ']':
answer = "NO"
break
return answer
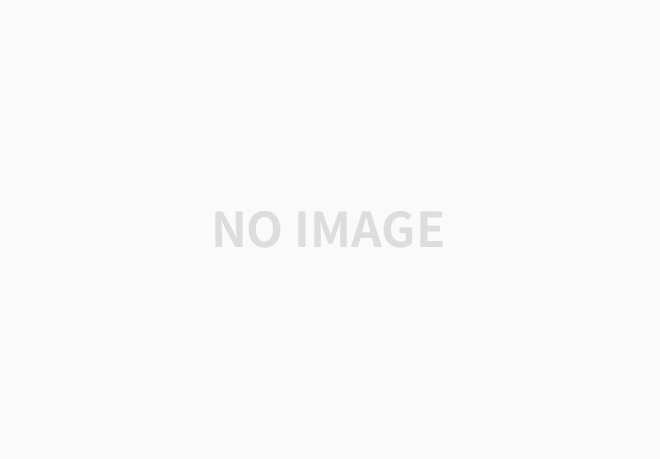
예제만 보면 양쪽을 비교하면서 원하는 모양의 괄호가 나오지 않으면 "NO"
원하는 모양의 괄호가 나오면 "YES"로 해야겠다 생각을 하게됩니다.
하지만 이렇게 코드를 짜서 제출하면 { ( ( [ ] ) [ ] ) [ ] } 와 같은 괄호는 찾을 수 없습니다.
그래서 우리는 이 괄호 문제를 풀때 스택을 사용하게됩니다.
두번째 코드
#!/bin/python3
import math
import os
import random
import re
import sys
# Complete the isBalanced function below.
def isBalanced(s):
stack = []
answer = "YES"
for i in range(len(s)):
if s[i] == '{':
stack.append('{')
elif s[i] == '(':
stack.append("(")
elif s[i] == '[':
stack.append("[")
elif s[i] == ']':
if len(stack) and stack[-1] == '[':
stack.pop()
else:
answer = "NO"
elif s[i] == ')':
if len(stack) and stack[-1] == '(':
stack.pop()
else:
answer = "NO"
elif s[i] == '}':
if len(stack) and stack[-1] == '{':
stack.pop()
else:
answer = "NO"
return answer
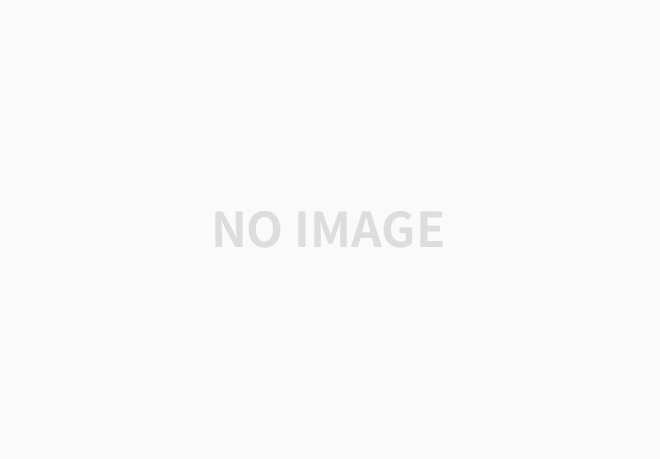
세번째 코드
#!/bin/python3
import math
import os
import random
import re
import sys
# Complete the isBalanced function below.
def isBalanced(s):
stack = []
answer = "YES"
open_bracket = ['{', '[', '(']
close_bracket = ['}', ']', ')']
for i in range(len(s)):
if s[i] in open_bracket:
stack.append(s[i])
elif s[i] in close_bracket:
index = close_bracket.index(s[i])
if len(stack) > 0 and stack[-1] == open_bracket[index]:
stack.pop()
else:
answer = "NO"
break
if len(stack) != 0:
answer = "NO"
return answer
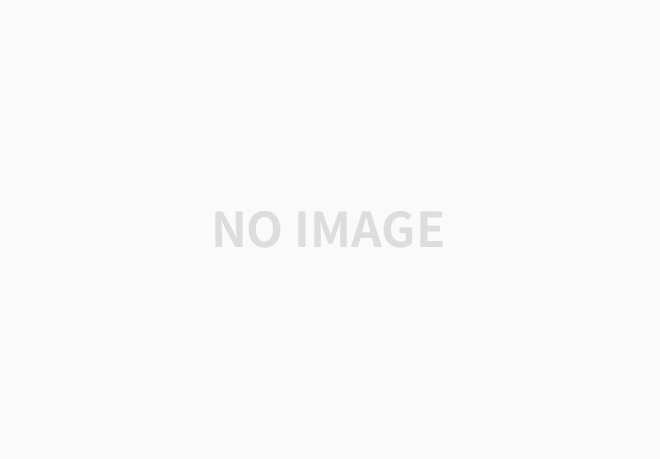
코드를 조금 줄이기위해서 괄호목록을 List로 만들어 비교했고 나머지 3개의 케이스를 통과시키기 위해서
stack이 비어있지 않을경우 "NO"를 return 하도록 했습니다.
오늘은 여기까지!
'Programming > 코딩 1일 1문제' 카테고리의 다른 글
[HackerRank] Hash Tables : Ransom Note (Python) (0) | 2020.03.22 |
---|---|
[SW_Expert_Academy] 4581번 문자열 재구성 프로젝트 (Python) (0) | 2020.03.21 |
[BaeKJoon] 11726번: 2xn타일링 (Python) (0) | 2020.03.19 |
실제 면접 코딩테스트 문제 (Python) (15) | 2020.03.18 |
[SW_Expert_Academy] 5688번 세제곱근을 찾아라 (Python) (0) | 2020.03.18 |